Adventures with Electronics is a father and son project to learn how to connect up and code 37 different electronic sensors using a Raspberry Pi Pico.
After setting up the software and firmware needed to write python code on a Raspberry Pi Pico, this is the second project in the adventure in electronics. It uses an 18B20 sensor to read the temperature and turns a motor on (connected to an ice skating lego minifigure) if the temperature is less than 22 ‘C.
About the sensor
The 18B20 sensor 3 pins and needs a resistor to be connected before it can be used. The version I have comes on a breakout board which already has this resistor and a built in LED which flashes when you read the sensor value.
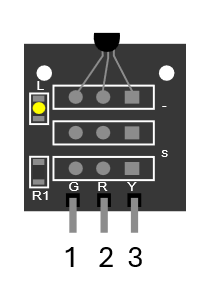
Pin number | Name | Description |
1 | G/GND | Ground (0v) |
2 | R/VCC | Power supply (3.3v or 5v) |
3 | Y/DQ | Data |
You can also get waterproof versions of this sensor. It’s worth noting that each sensor has a unique ID number so you can connect as many as you like to the same pin on the Pico and read the values separately.
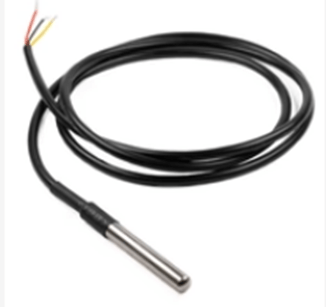
The circuit
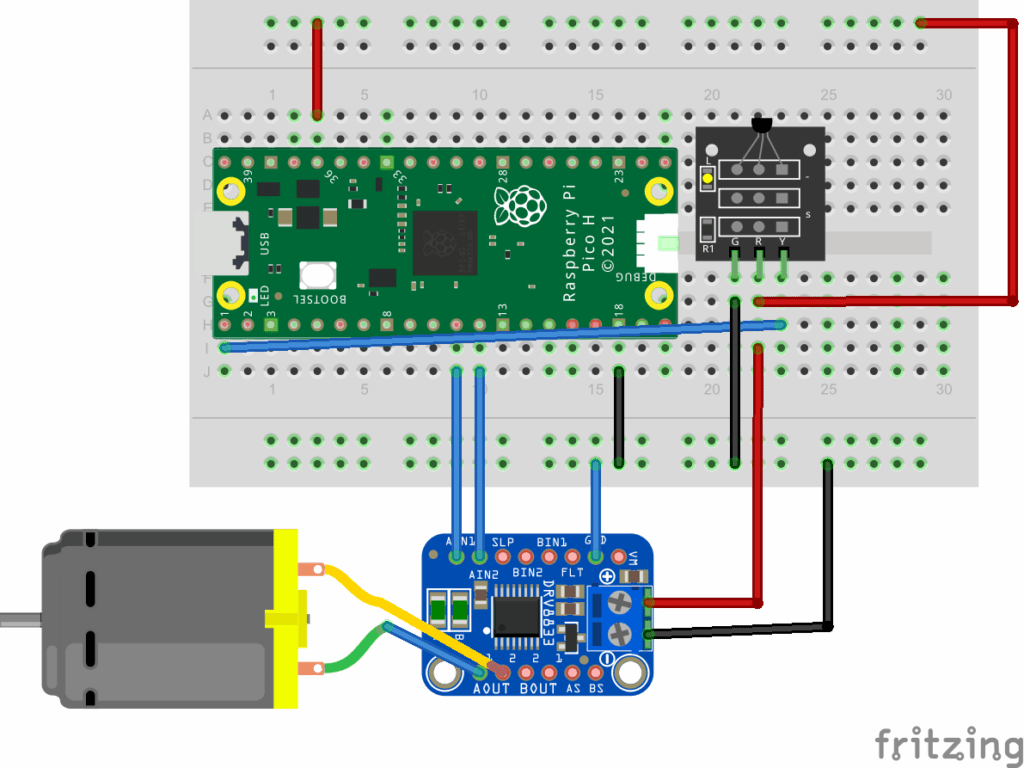
The DRV8833 motor driver breakout shown is integrated into the pico explorer board but you can get one separately here.
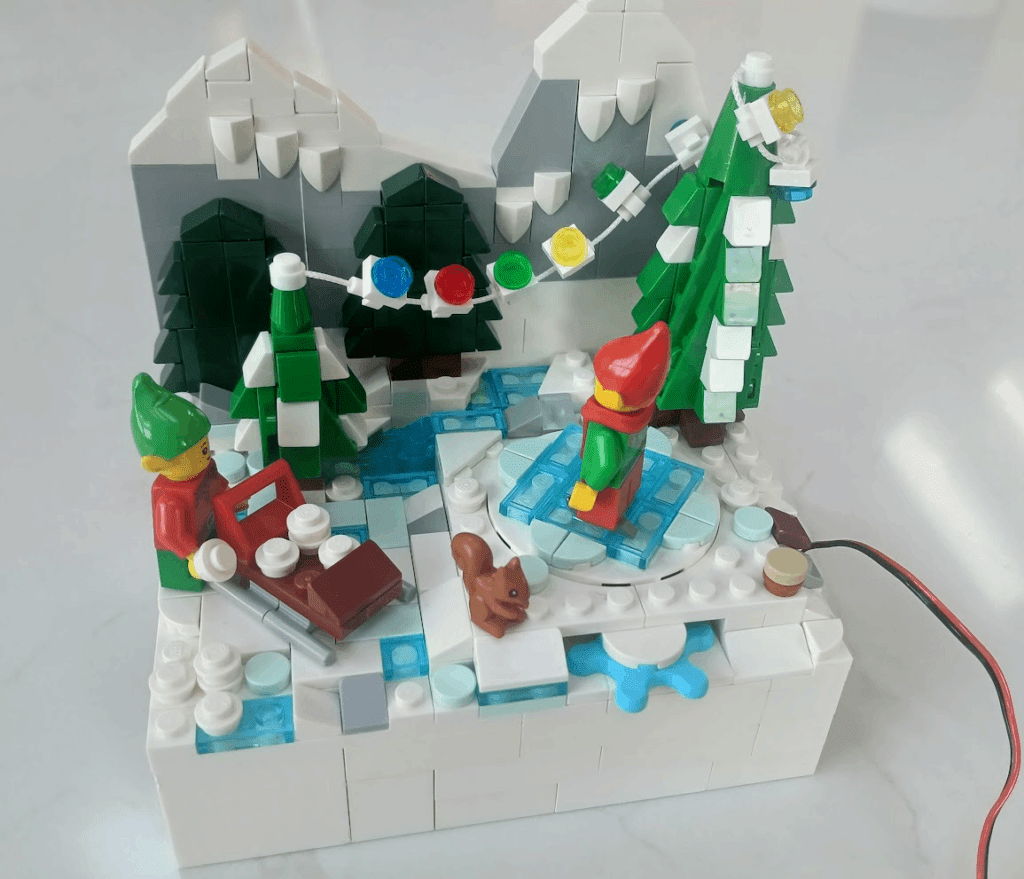
The motor is a Geekservo Lego Compatible motor connected to a Lego scene with an ice skating elf (but any other scene will do!)
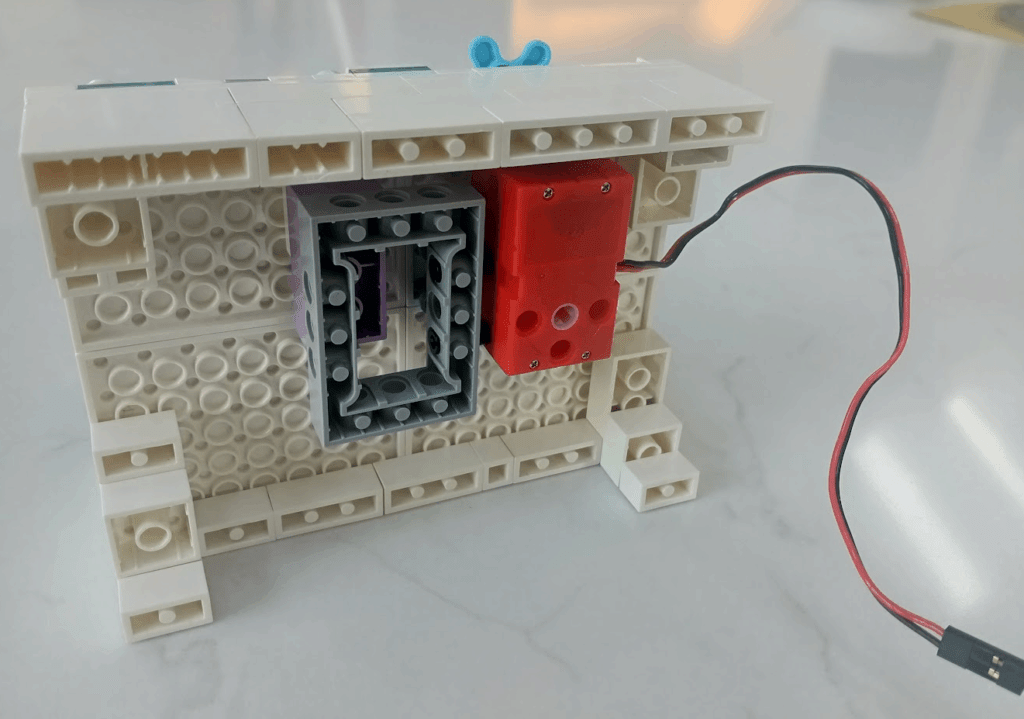
The code
The buttons next to the pico explorer board can be used to set the motor speed in manual mode, or it will automatically switch on the motor if the temperature is cool enough to ice skate.
"""
Adventures in Electronics
* Firmware:
- CircuitPython v9.2.1
* Hardware:
- Raspberry pi pico in pico explorer board
- 18B20 temperature sensor
Pin1 (GND/G => GND)
Pin2 (VDD/R => 3v3)
Pin3 (DQ/Y => GP0)
* Description:
Reads temperature ('C) every second and displays on screen
Spins motor 1 if temperature is less than 22'C.
* Buttons:
A: auto mode (spins if temperature is less than 22'C)
B: manual mode (use X and Y to change speed)
X: increase speed by .1 in manual mode
Y: decrease speed by .1 in manual mode
"""
import picoexplorer
import board
import time
import adafruit_ds18x20
from adafruit_onewire.bus import OneWireBus
ow_bus = OneWireBus(board.GP0)
mode = "auto"
speed = 0.0
# scan for all sensors (you can have lots connected to the same pin)
devices = ow_bus.scan()
if len(devices) == 0:
print("No devices found")
else:
id = "".join([hex(i)[2:] for i in devices[0].rom])
print("ROM = {} \tFamily = 0x{:02x}".format(id, devices[0].family_code))
picoexplorer.init()
# only connect to the first device found
ds18b20 = adafruit_ds18x20.DS18X20(ow_bus, devices[0])
while True:
# read temperature
temperature_c = ds18b20.temperature
t = 'Temp: {0:0.3f} °C'.format(temperature_c)
print(t)
picoexplorer.set_line(3, t)
# switch on the motor if temperature is below 22'
if mode == "auto":
if temperature_c < 22:
speed = .3
else:
speed = 0
if mode == "manual":
if not picoexplorer.buttons["X"].value:
speed += 0.1
if not picoexplorer.buttons["Y"].value:
speed -= 0.1
if not picoexplorer.buttons["A"].value:
mode = "auto"
if not picoexplorer.buttons["B"].value:
mode = "manual"
if speed > 1:
speed = 1
if speed < -1:
speed = -1
picoexplorer.motors[0].throttle = speed
picoexplorer.set_line(4, "{} speed: {:.1}".format(mode, speed))
# No faster than 10Hz
time.sleep(.1)
Code language: PHP (php)
Link to code (including the picoexplorer module and other required libraries) here.
There’s also some micropython code just to read from the sensor here.