This beginners tutorial talks you through how to get started displaying and manipulating images on a BBC micro:bit.
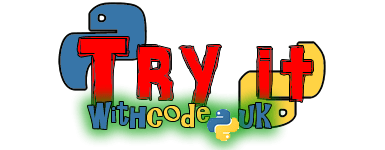
Try the code: python microbit basics: How to display images
Run the python code below in the micro:bit simulator to see three different ways of showing an image:
The easiest way to display an image is to use a built in image such as Image.HAPPY
from microbit import * # show built in images display.show(Image.HAPPY) sleep(1000) display.show(Image.SAD) sleep(1000) # define your own image on separate lines i = Image("00000:" "22222:" "44444:" "66666:" "88888") display.show(i) sleep(1000) # define your own image on one line i = Image("88888:66666:44444:22222:00000") display.show(i)
Lines 4 and 6 show you how to display a built in image. Thesleep(1000) lines pause the program for 1000 milliseconds (1 second) so that the images are on the screen long enough for you to see them. You can find a list of built in images on the documentation page.
Once you’ve tried out some of the built in images, it’s more fun to create your own images:
from microbit import * # show built in images display.show(Image.HAPPY) sleep(1000) display.show(Image.SAD) sleep(1000) # define your own image on separate lines i = Image("00000:" "22222:" "44444:" "66666:" "88888") display.show(i) sleep(1000) # define your own image on one line i = Image("88888:66666:44444:22222:00000") display.show(i)
Lines 10-14 creates a new Image object called i and sets the brightness of each of the LEDs according to the numbers.
0 for each LED means that it is switched off and 9 means it’s fully on.
You can’t actually set the brightness of a LED by dimming it like a lightbulb, but you can fool the human eye into thinking the brightness of an LED has been changed by switching it on and off very quickly for varying amounts of time. Python does this for you behind the scenes.
from microbit import * # show built in images display.show(Image.HAPPY) sleep(1000) display.show(Image.SAD) sleep(1000) # define your own image on separate lines i = Image("00000:" "22222:" "44444:" "66666:" "88888") display.show(i) sleep(1000) # define your own image on one line i = Image("88888:66666:44444:22222:00000") display.show(i)
Line 19 looks a little more confusing but it’s just a more compact way of defining an image than lines 10-14. Each row of the image is separated by a colon (:) and the brightness of each LED is represented by a number from 0 (off) to 9 (full brightness)