The Theory:
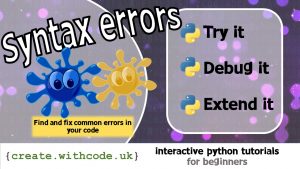
Syntax errors: Find and fix common errors in your code
By now you’ve probably discovered that the smallest mistake in your python code can stop the whole thing from running.
There are three types of error: syntax errors, runtime errors and logical errors. With practice, you’ll soon pick up experience in debugging all three types of error but for now we’ll focus on syntax errors.
Syntax means structure and rules
You’ll get a syntax error when you break the rules of the programming language (e.g. forget a bracket or “)
Syntax errors prevent your code from running at all.
Even if your code is full of syntax errors you’ll only see an error message for the first one.
You’ll know you’ve got a syntax error because an error message will appear as soon as you try to run your code. These error messages may look intimidating, but they always give you a clue which line to start looking at to find the problem.
The line number on an error message might not be exactly where the error is.
For example, if you try to run the code below by pressing Ctrl + Enter
you’ll get an error message saying there’s a problem with line 2:
Actually, there’s a )
missing from the end of line 1. Python tries to look for it on line 2 and can’t find it so that’s why it gets confused with the line numbers.
Tip: Always look for the line number in an error message. It means there’s probably something wrong with that line or the last line of code above it (ignoring comments or blank lines)
Once you’ve tracked down where the error is, work your way through the following steps:
- Ask yourself what you want the code to do
Sometimes just taking a step back and explaining the process to yourself or someone else is enough for you to spot what you’ve done wrong.
- Check that quotation marks and brackets are all balanced.
Balancing means whenever you open a set of brackets with
(
you’ll need to close them with)
somewhere on the same line or section of code. It’s the same with"
and[
]
. - Check your spelling and capitalisation.
Python can’t cope with spelling mistakes. It thinks
Print
is different toprint
so sometimes it helps to increase the code font size (Ctrl +
) and read it through carefully. - Check indentation.
Indentation means how far the code is spaced from the left side of the screen. Code should always be lined up perfectly with the line above unless there’s a good reason for it not to (e.g. starting or ending a loop or function).
- Google the error message
Once you’ve tried to fix your code yourself, it’s time to copy the error message and paste it into a search engine online or ask someone else for help.
On the next page you’ll get some code examples that you can try out for yourself.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Amazing! You are helping me to feel more confident in teaching Python! Thank you, and keep up the great work!