Learning to understand, write and debug python programs can be hard work, especially if python is the first programming language you’re learning.
This set of resources is aimed a beginners who want a quick reference guide and a variety of programming challenges of varying difficulty where you get instant feedback without having to sign up or pay to get going.
This page contains links to a set of self-marking python activities that let you track your progress and download and / or print off a PDF certificate for each python skill.
The aim is to publish a complete set of 20 activities but it’s a work in progress so do let me know if you spot any mistakes or can think of a way to improve any of the activities.
Each activity has four sections:
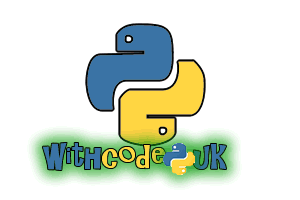
- Theory: Learn the key words and concepts
- Try It: Look at and run some working example code
- Debug It: Practise debugging some common errors
- Extend It: Adapt and extend code with ideas for bigger projects
Published Python for Beginners Activities:
Here’s the activities I’ve published so far:
01: Output
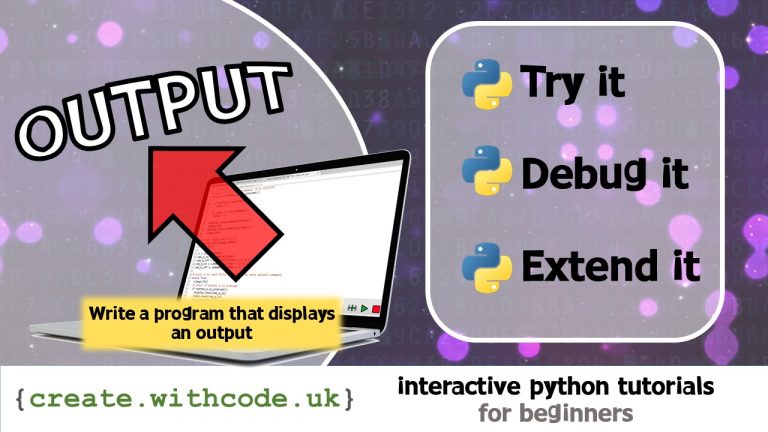
02: Input
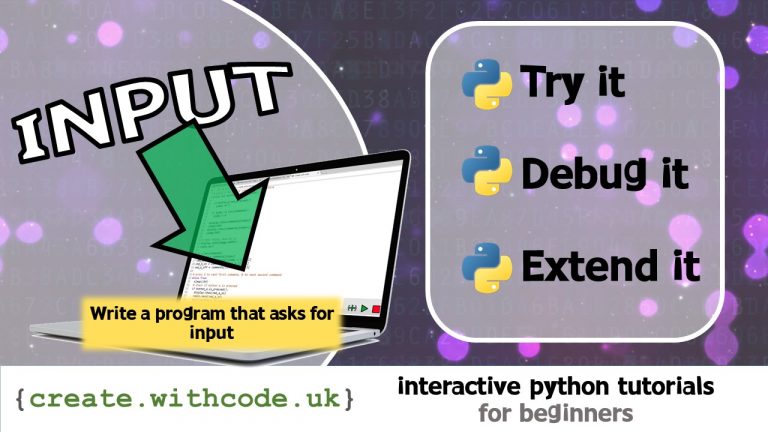
03: Syntax Errors
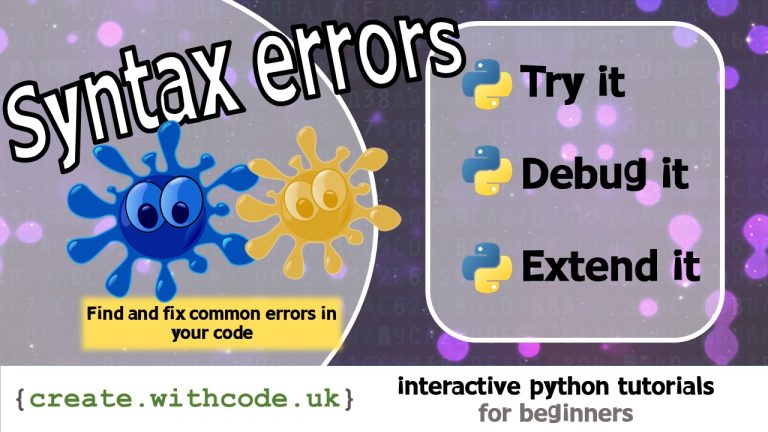
04: Variables
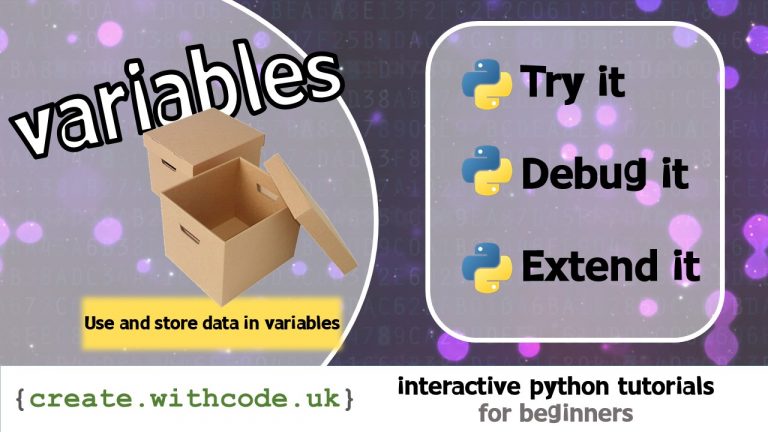
05: Naming Conventions
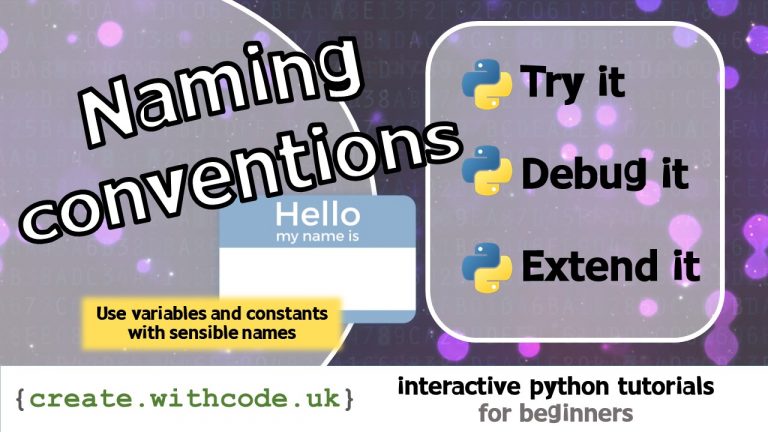
06: Data Types
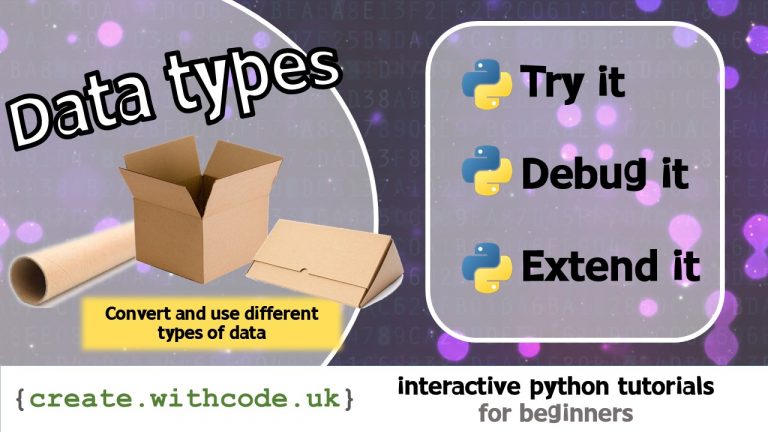
07: Runtime Errors
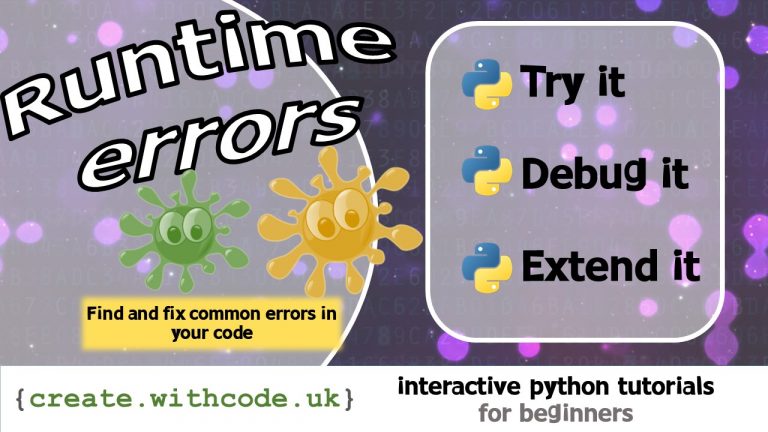
08: Sequence
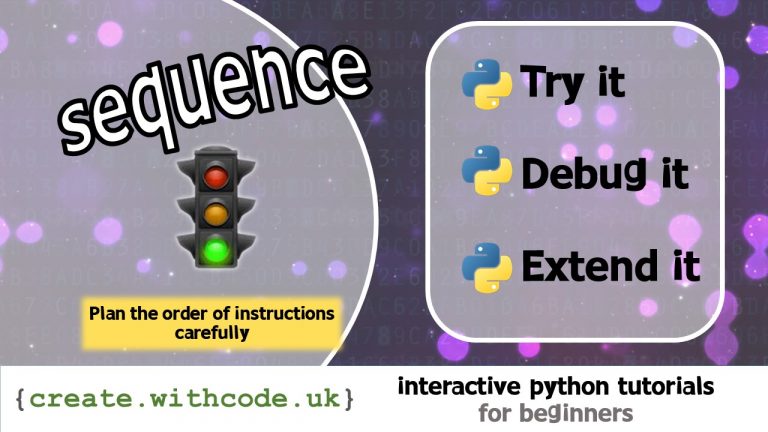
09: Selection
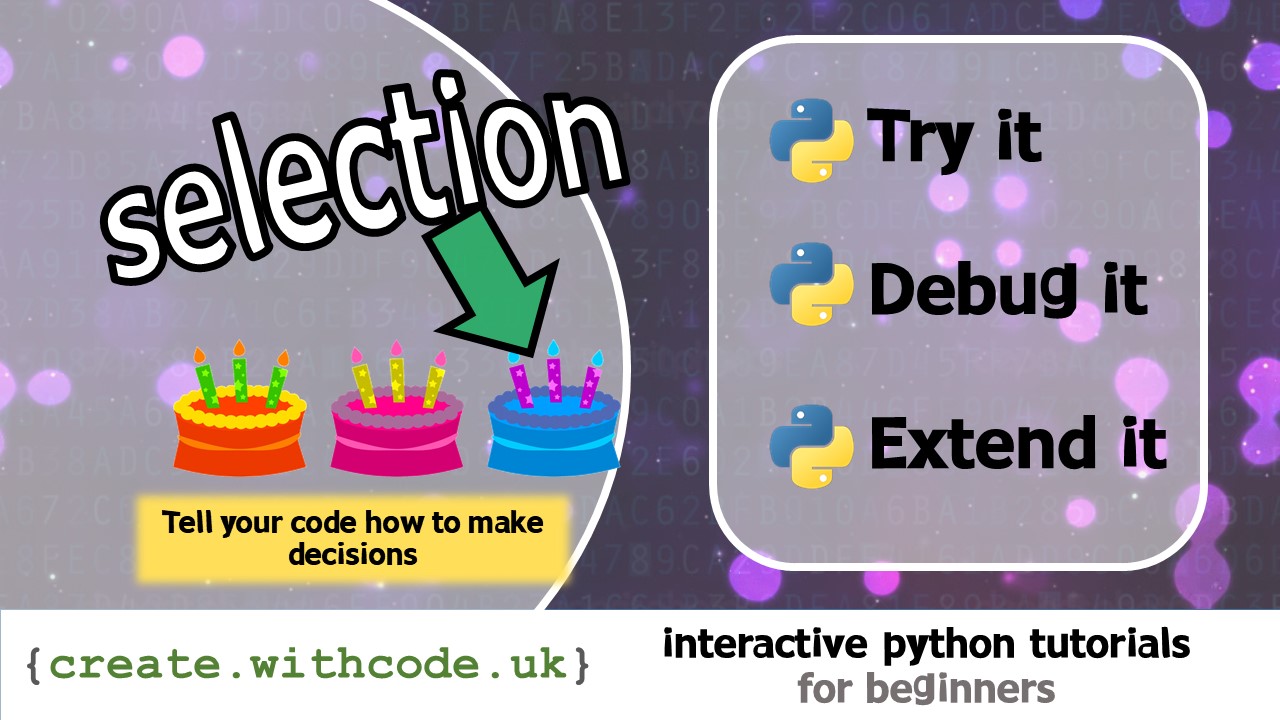
10: Logic errors
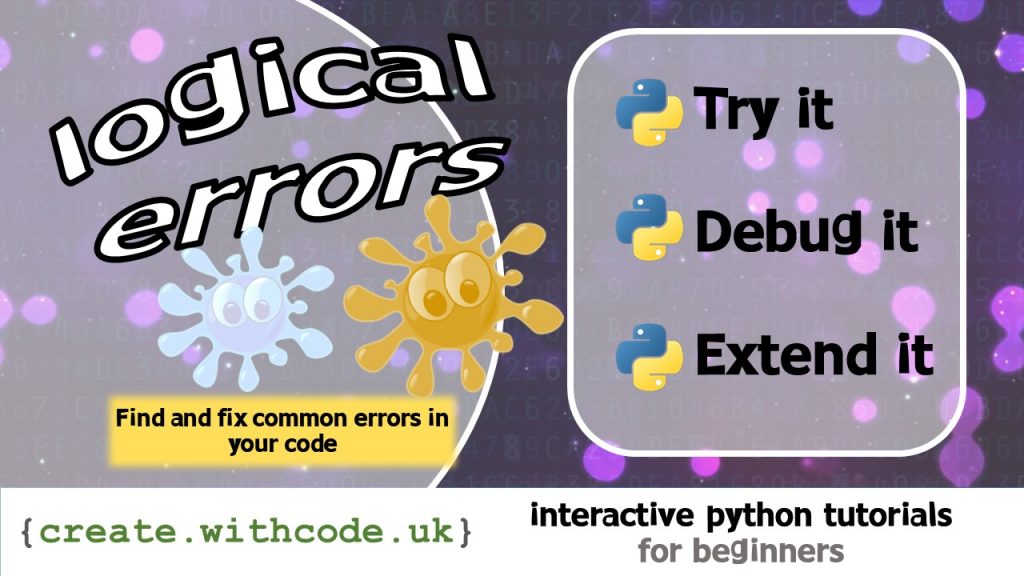
11: Lists
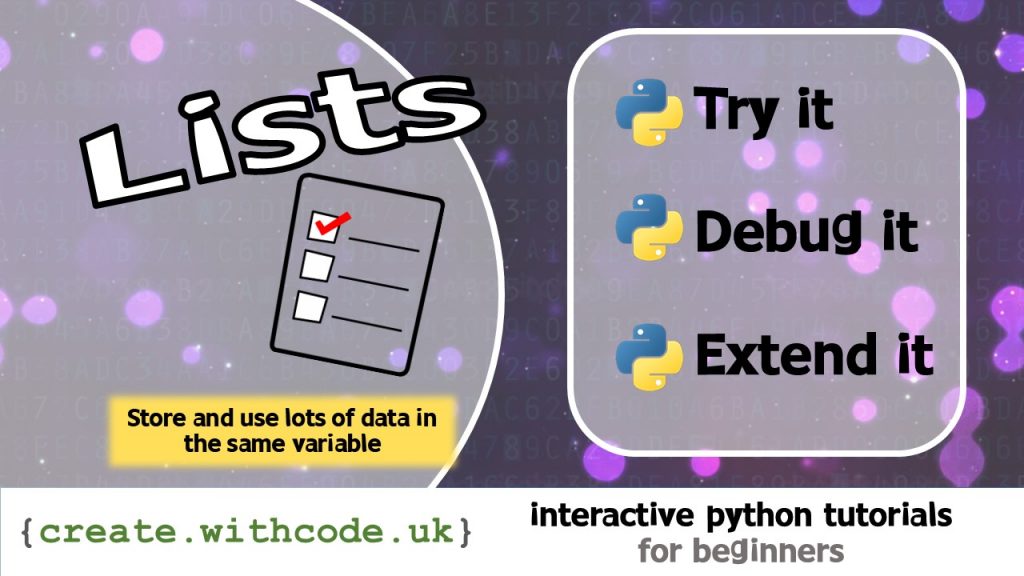
12: Iteration
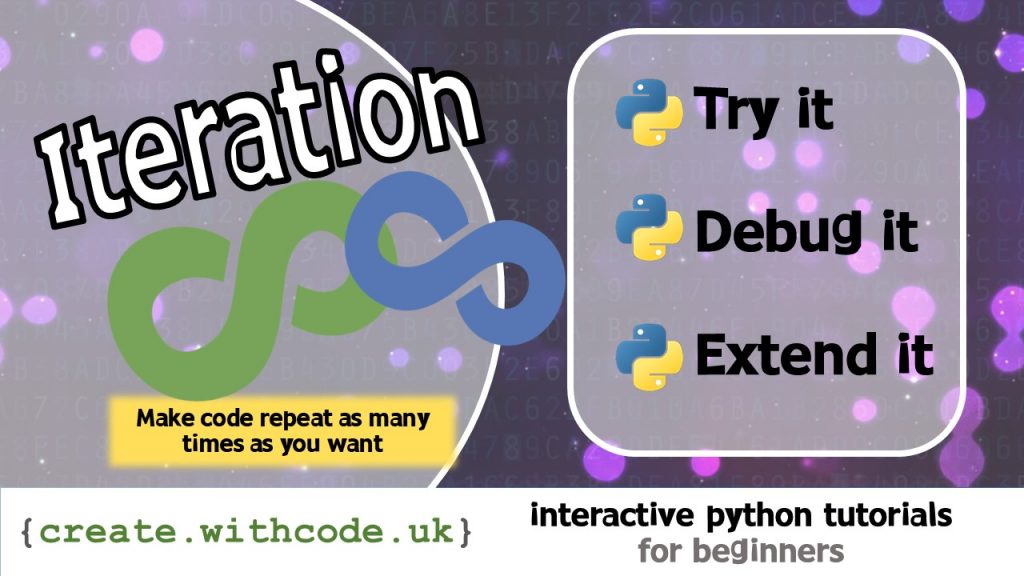
13: Writing to files
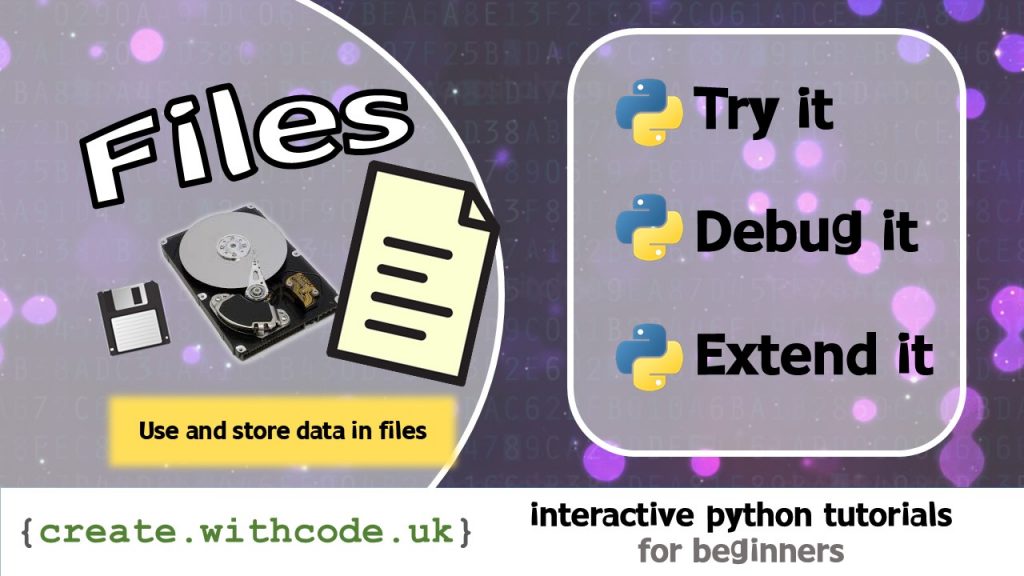
14: Reading from files
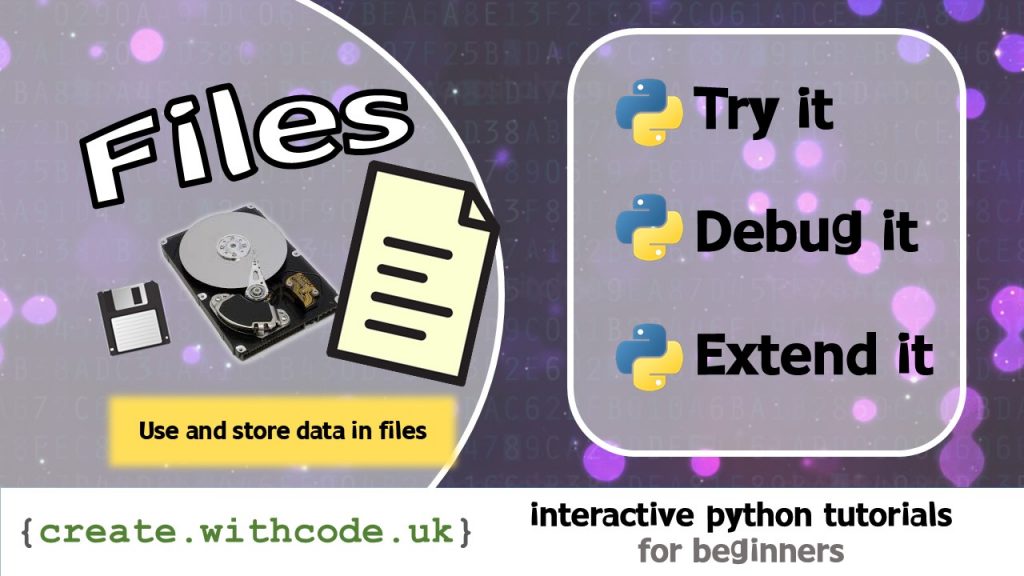
15: Error handling
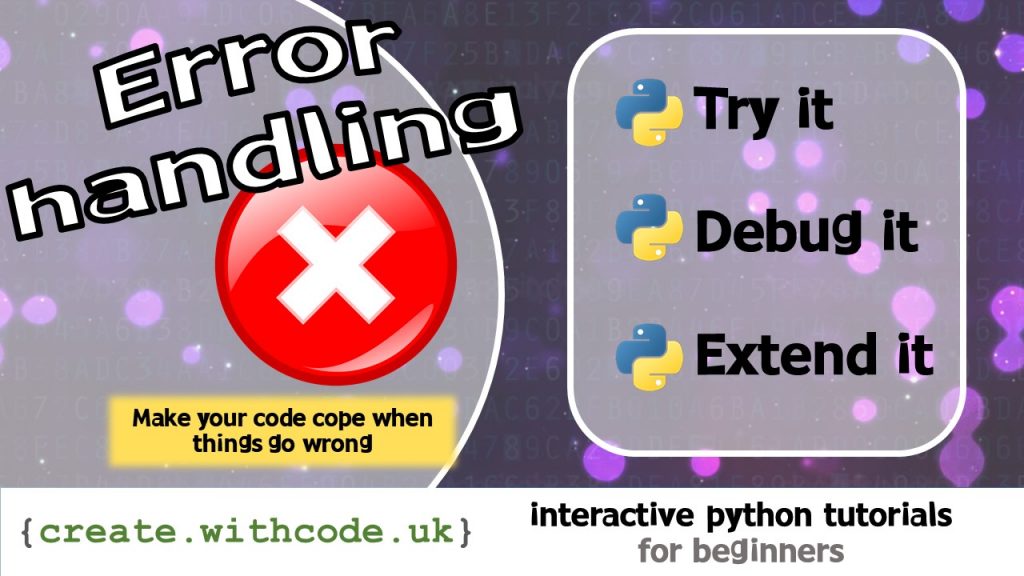
16: Procedures
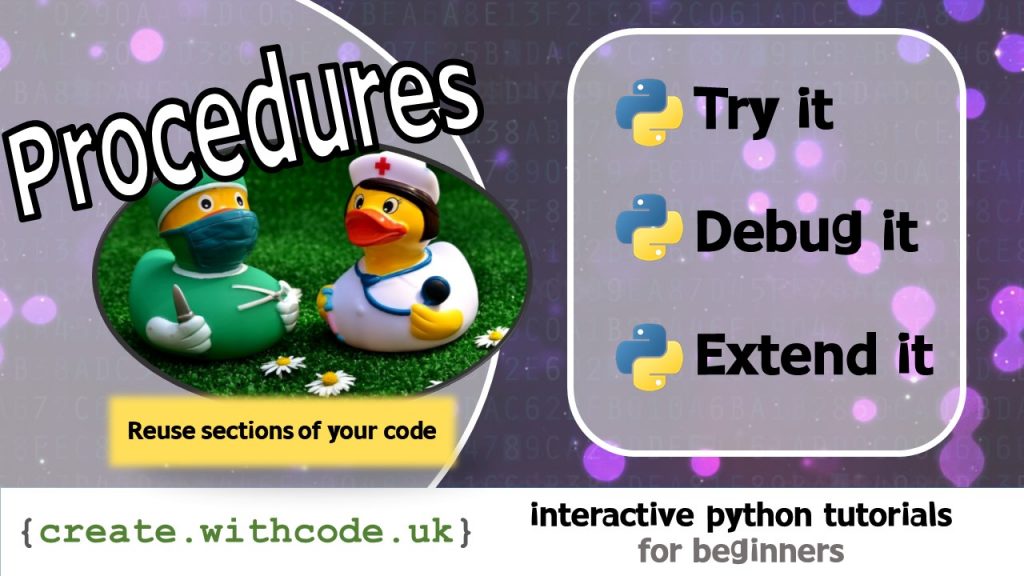
17: Functions
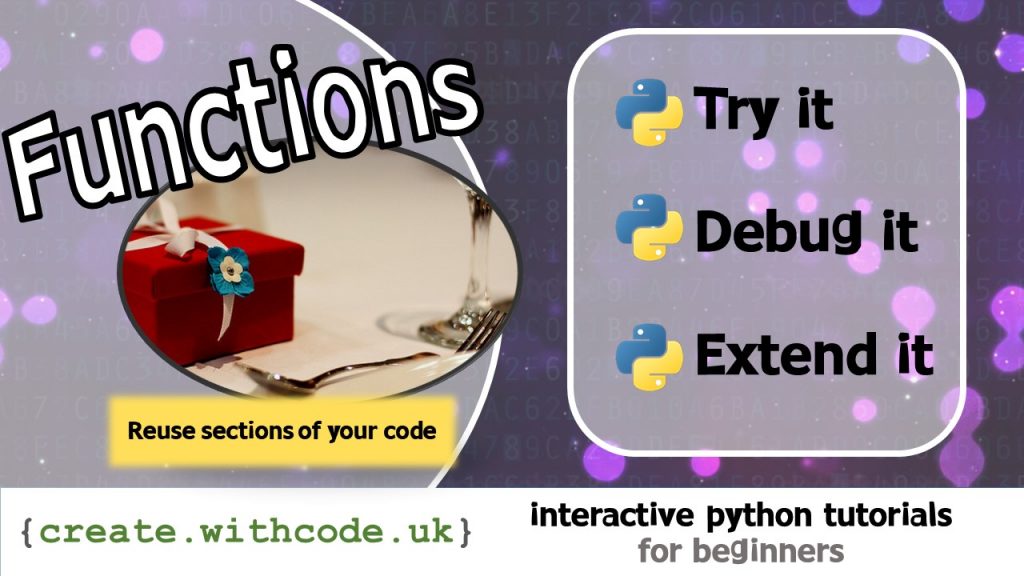
18: Parameters
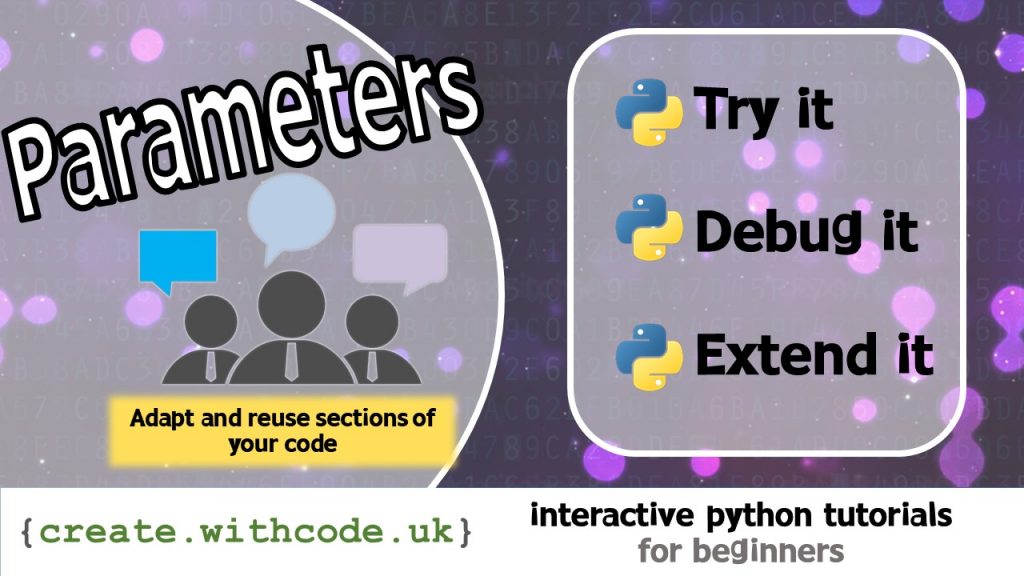
19: Optimisation
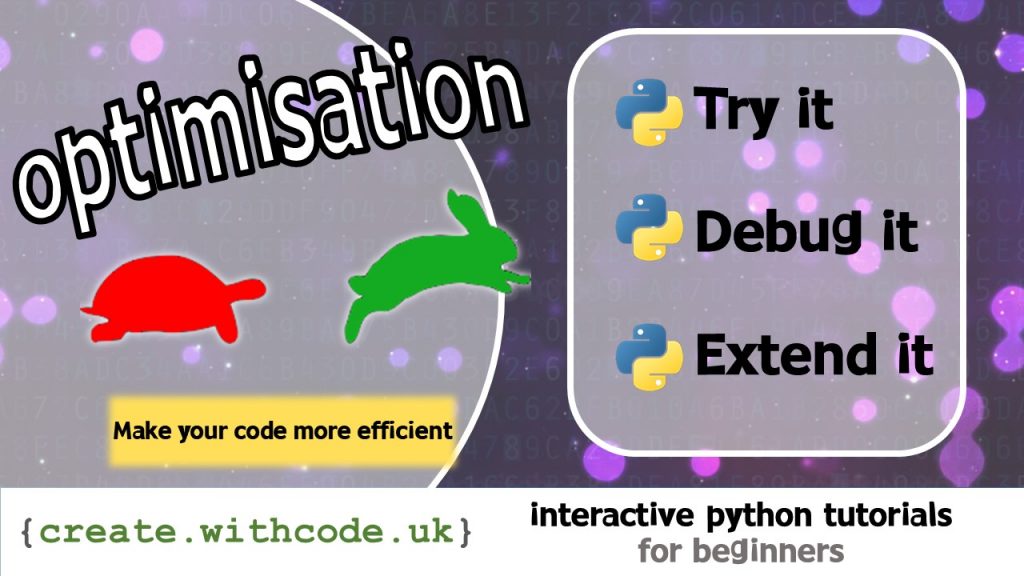
On the next page you’ll see a list of all of the activities that are planned to be published in the future.
Hi
Really nice resource, love the accessibility, layout, tasks, everything really.
Have spotted a few typos: your self/yourself …. it’s/its….. a/at
More than happy to point them out if you let me know where and how.
Thanks Austin. Would really appreciate the quality control feedback on typos-thank you. Just comment like this wherever you see them. Thanks very much 😀
Brilliant resource. Thank you for sharing. The kids love the certificate at the end and it’s a great way to track progress.
Great resources! Thank you for doing this! Looking forward to the next ones!