The Theory:
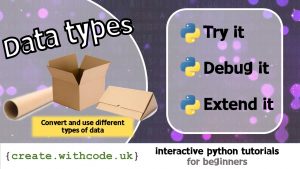
We’ve already learnt how you can use variables to store data so that your code can process and display it, but so far we’ve only looked at text data.
The next step is to look at how to deal with numbers as well as text.
Before, we said that variables are like boxes used to store stuff. We said that variables have a name (like the box’s label) and a value (like what’s inside the box).
Variables also have a type which is like the shape of the box. There are three simple data types you should be aware of:
Integer or int: a whole number like
1
,2
or3
but not1.2
or3.6
Real or Float: a number that has a decimal point like
1.123
or3.14
String: text which can contain characters, punctuation or numbers like
"hello"
or"45 degrees"
or even"45"
but not45
It’s important to note that python thinks the string "45"
is different from the int 45
For example, when you add integers together, you add the number values.
45 + 45
is90
However, when you add strings together, you join them one after the other:
"45" + "45"
is"4545"
Joining strings together is called concatenation.
Sometimes it’s useful to convert from one type to another.
For example, input("How old are you")
will ask the user a question for which you’d expect them to type a number (e.g. 15
). However, the value that you get back from input is a string (e.g. "15"
). If you want to do any calculations using that value, you’ll need to convert it to an integer first.
Converting to integers:
int("2")
andint(2.1)
both return the integer value2
Converting to strings:
str(2)
andstr(1 + 1)
both return the string value"2"
Converting to real numbers:
float(2)
orfloat("2")
both return the real number2.0
Converting from one data type to another is called casting.
You can use any combination of variables, constants and values inside the brackets of these functions.
Key point: use
int(...)
,str(...)
andfloat(...)
functions to cast data to integers (whole numbers), strings (text) and reals (floating point decimal numbers).
On the next page you’ll get some code examples that you can try out for yourself.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Trackbacks/Pingbacks