The Theory:
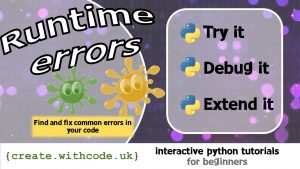
Debugging your code can be slow and frustrating to begin with but it’s very satisfying when you work out how to fix something for yourself.
There are three types of errors you’ll come across with your code: Syntax errors, run time errors and logical errors.
Syntax errors happen when you break the rules of the language like accidentally missing out a
"
or)
. Syntax errors stop your code from running at all.Runtime errors happen when your code tries to do something that’s impossible like trying to convert the text
"potato"
into an integer number data type. Runtime errors make your program crash whilst it’s trying to do the thing that’s impossible.Logical errors happen when your code does what you told it to do, but not what you wanted it to do, like adding two numbers instead of subtracting them. Logical errors might not crash your program but will cause it to do the wrong thing.
The easiest of the three types of errors to find are syntax errors because you get an error message appearing as soon as you try to run your code.
Finding and fixing runtime errors is easier than debugging logical errors because you will at least get an error message that usually tells you which line of code that is broken.
However, sometimes you need to test your code thoroughly with a wide range of input data in order to detect the runtime error.
For example: When you’re testing a program that asks you to type in a number, you don’t just test it by typing in a sensible number but you see what happens if you put numbers in that are deliberately too small, too big or not even numbers at all.
Once you’ve found the line of code that causes the runtime error, you can surround that line with try:
and except:
in order to tell python how to react to the error without crashing.
If you see try:
in some python code, it means that a runtime error could potentially rear it’s ugly head in the code that’s indented under that line, but python will attempt to run it anyway.
If it all goes wrong, python will skip to the line that says except:
and run the code indented under that line instead of crashing.
The aim is to be able to write a program that runs without ever crashing, even if the user running the code is an elite hacker trying to break it, or a newbie technophobe who doesn’t really know what they’re doing.
Code that can cope with anything without crashing is called robust code.
On the next page you’ll get some code examples that you can try out for yourself.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
For some reason when I save my code it doesn’t give me a new url to use, do you know why this is?
Hi Lucas. Sorry it’s not giving you a new url.
When you change the code in one of these tracked activities it will automatically save your changes just on your computer in a cookie so that if you refresh your page you don’t have to start all over again. If you want a new URL you’ll have to press Ctrl+S. You may have to tick the ‘I’m not a robot’ box if that comes up on screen. This is irritating but it limits people exploiting the fact that anyone can save without having to log in. If you’re still having problems, reply here and I’ll email you.