Debug it
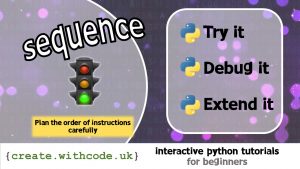
Below is some code that has been deliberately broken so that you can fix it. You don’t need to change any of the actual code, just rearrange the lines into a different order so that the sequence of instructions does what you need it to.
Challenges
- Define the turtle object
t
before using it
Line 3 imports the turtle module which lets python know how to do drawing, but at the moment, the code tries to use a turtle object
t
before it’s been defined on line 21.Move line 21 up, so that it appears before any other mention of
t
- Rearrange lines 7-11 to draw a triangle
If you’ve changed the order of your code, lines 7-11 were originally as follows:
# draw a black triangle
t.right(120)
t.forward(50)
t.forward(50)
t.right(120)
t.forward(50)
Think about the steps needed to draw a triangle then change the order of these lines of code.
- Rearrange lines 14-18 to get rid of the line between the triangle and the text
Lines 14-18 were originally like this:
# write "Hello" in red at the top of the screen
t.color("red")
t.pendown()
t.goto(0,100)
t.penup()
t.write("Hello",font=("Arial", 20))
What’s currently happening is that the turtle jumps to the top of the screen whilst the red pen is down on the screen so it draws a line. Change the order of the instructions so it lifts the pen up before moving.
On the next page you’ll get some ideas for projects that work with sequences of instructions.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Trackbacks/Pingbacks