Try it:
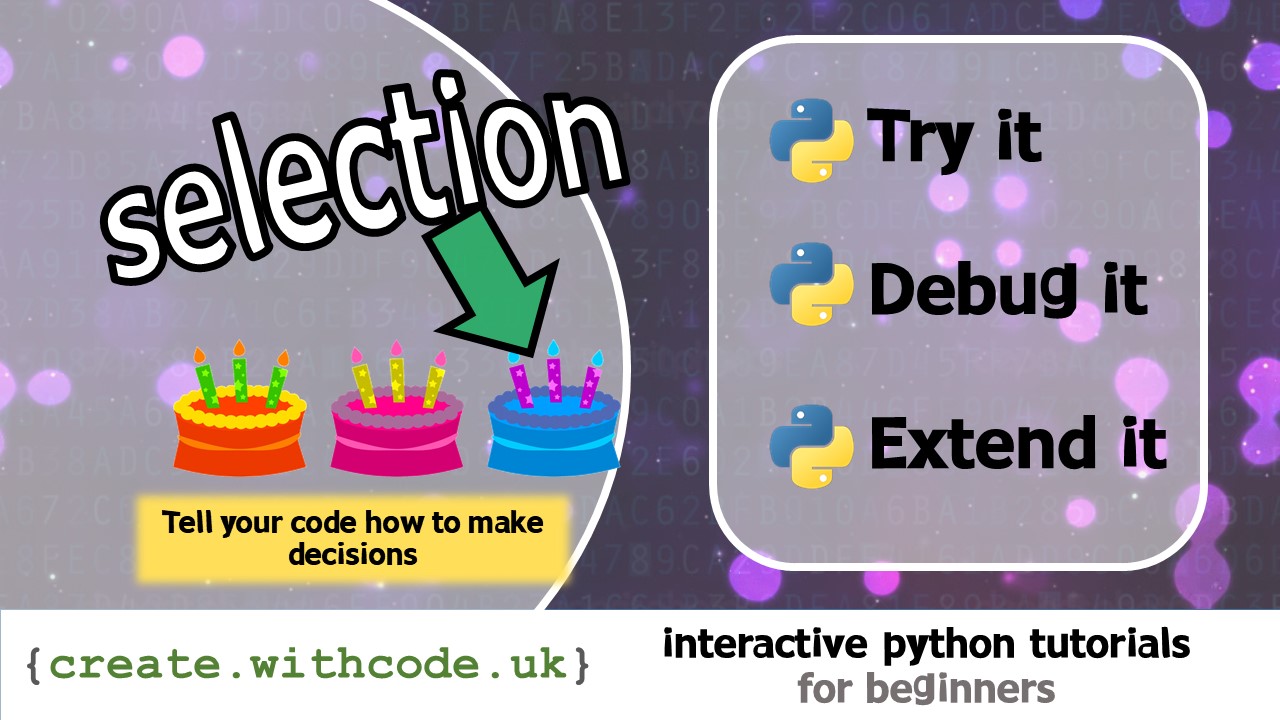
If you run the code below you should see a program that reacts and responds to user input:
Press Ctrl + Enter
to run the code.
This code will ask your name. It uses selection to say Snap!
if you type in “chatty
” which it thinks is its name too.
The code them asks how old you are. It uses selection again to react to your age.
Challenges:
Start by running the code and testing it with a variety of different answers to the questions.
- Change it so that if the user leaves their name blank the program will say “
Don't be shy
“
You’ll need another if statement to check if name is equal to an empty string (
""
).Be careful with indentation: your if statement should be all the way to the left but the line underneath should be indented to the right
- Extend it so that if the user says they’re over
100
years old, the program says “I don't believe you
“
Find the code that says
if age < 5:
and the twoprint
lines that are indented underneath. These two lines will only run if the user say’s they’re under 5 years old.Under these lines but above the line that says
else:
add a line that sayselif age > 100:
elif
means “Else, if”. You can use it under anyif
statement as long as it’s perfectly lined up (in terms of indentation from the left of the screen) - Adapt the code so that even if the user says their name is
CHATTY
orChatty
it still says “Snap!
…”
You can convert strings to lowercase letters by putting
.lower()
after the name of the variable that stores a string. You can also use.upper()
to convert to uppercase letters.Find the line that checks if the variable called
name
stores a string that is equal to “chatty
” and changename
toname.lower()
On the next page you’ll get some code with some common errors that you can use to practise debugging.
KPRIDE
KPRIDE stands for Keywords, Predict, Run, Investigate, Debug and Extend and it’s a way of helping you explore and understand python code. Click on the image below for a set of KPRIDE activities for this python skill.
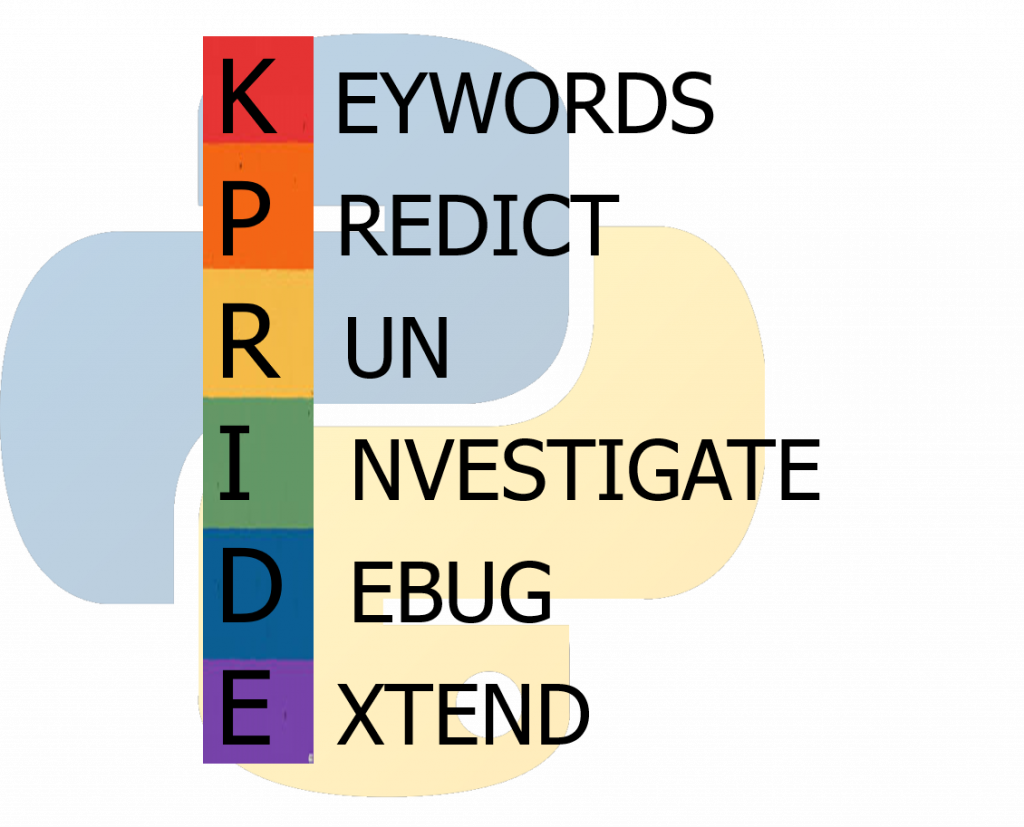
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Nice post! Thanks for sharing information.