The Theory:
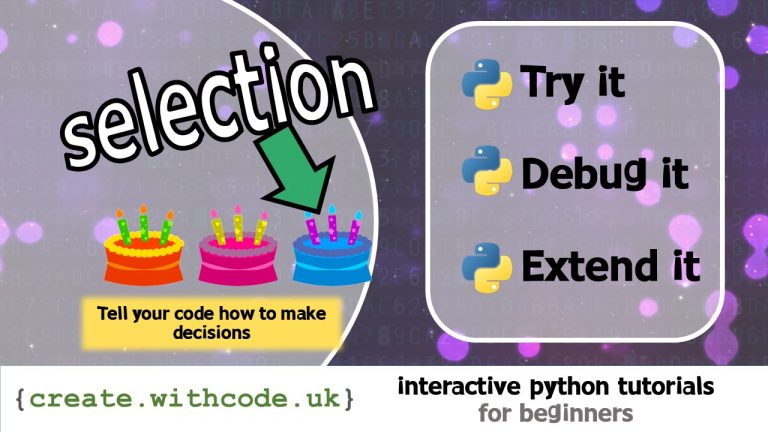
Humans are brilliant at making decisions.
The average person makes over 200 decisions every day… just about their food!
Wansink, Brian and Jeffrey Sobal (2007), “Mindless Eating: The 200 Daily Food Decisions We Overlook,” Environment and Behavior 39:1, 106-123
Your brain is constantly receiving and processing information from the world and deciding how you should react in response.
Computers can be told how to make decisions using selection.
A key skill for a good programmer is working out which logical decisions their programs will have to make.
In python code, selection is usually where you use if to decide which instructions to run next, based on a boolean expression
Think of a boolean expression as a question that can only be answered by either
yes
(True
) orno
(False
)
So, the following expressions are examples of boolean expressions:
# Does the variable called hungry contain "yes"?
hungry == "yes"
# Does the variable called age?
age > 13
# is the price less than the maximum I can afford?
price < budget
Code language: PHP (php)
But the following are not boolean expressions:
# This expression evaluates to a string, not a boolean
"hello " + name
# This expression evaluates to an integer not a boolean
score + 1
Code language: PHP (php)
Once you’ve decided what decision you want your code to make, you can use if to get python to only run certain code if your boolean expression evaluates to True
.
For example:
if hungry == "yes":
print("Eat some cake")
Code language: PHP (php)
This code will only display “Eat some cake” if the variable called
hungry
contains the string value"yes"
Here are four top tips:
- Use
==
rather than=
if you want to check two values are the same
A single
=
will set the value of a variable but if you want to check to see if two values are equal then you need to use==
- Lines starting with
if
always end with:
This tells python that the line(s) underneath will only run if the boolean expression (question) you’ve written evaluates to (equals)
True
- Indent the lines under your
if
statement
In python, whenever you see a
:
at the end of a line it means that the next line (or lines) should be indented (moved across to the right).Any lines that are indented will only run if the boolean expression in the if statement evaluates to
True
On the next page you’ll get some code examples that you can try out for yourself.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Nice post! Thanks for sharing information.