The Theory:
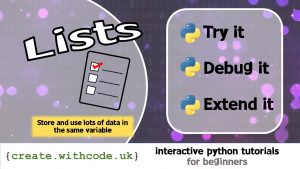
A list is a data structure which can store lots of different values in a specific order.
Creating a list
You can create an empty list to_do_list = []
or you can create lists that start with values already stored inside to_do_list = ['learn python', 'eat cake']
In python, a list can store pretty much anything. You can have lists of numbers: prime_numbers = [2, 5, 7, 11]
You can have lists of strings: colours = ["red, "orange", "yellow", "green", "blue", "indigo", "violet"]
And you can have lists which contain a mixture of different data types: my_list = [True, 1, 2, 3, "potato", 2.59]
List Indexing
The position of an item within a list is called the index.
List indexing starts at 0 not 1. This often catches people out, because what we think of as the 1st item in a list python calls the item at index 0
For example:
colours = ["red, "orange", "yellow", "green", "blue", "indigo", "violet"]
This creates a list called
colours
. There are 7 values stored in the list. The value at index 0 is"red"
and the value at index 5 is"indigo"
You can access or change these values using square brackets:
print(colours[0])
would display the first colour red
and colours[5] = "purple"
would replace "indigo"
with "purple"
Rather bizarrely (but very usefully), you can also use negative numbers as an index:
colours[-1]
means the last value in the list called colours, which is "violet"
and colours[-3]
would be "blue"
because it’s the third from the end.
Adding and removing values
Once you’ve made a list you can add data to it using append
:
to_do_list = []
to_do_list.append("get up)
to_do_list.append("eat breakfast")
is the same as:
to_do_list = ["get up", "eat breakfast"]
Note that the order that you add items to a list is important. Append means add on to the end, so your new values get added after any existing values in the list
You can also remove values from a list:
to_do_list.remove("get up")
Or you can remove the value at a particular index using pop
:
to_do_list.pop()
will remove the last item in the list or to_do_list.pop(0)
removes the item at index 0`
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.