The Theory:
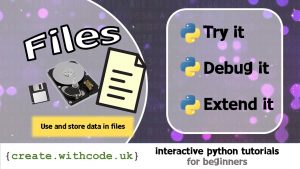
Computers have two types of memory to store data: primary storage and secondary storage.
Primary storage (e.g. Random Access Memory) is very fast but only stores data whilst the power is switched on.
Secondary storage (e.g. Hard Disk Drive) is slower but data is stored even when the computer is switched off.
Writing to files
Data on secondary storage is organised into files. Users and programs can save data into files so that they can access it again later.
Often programs output data into a file so that it can be read either by the user or by another program.
When you open a file in python you have to choose which access mode you want:
Write mode (
"w"
) means when you write data to the file you’ll replace anything that’s already in that fileAppend mode (
"a"
) means you’ll save any new data on to the end of the file
If you open
a file as in the example above, you have to be careful to close it again afterwards. A better way of doing this is to use the with
keyword which will automatically close the file at the end of the indented block of code:
Once you have opened a file you can write to it as many times as you like using the write
procedure:
The data passed to
write
needs to be a string which is why the example above wraps the numbercountdown
with thestr
function on line 6.The
\n
on line 6 means write a new line character to the file (otherwise all the numbers would all be on the same line)
Appending to a file
If you change the write mode from "w"
to "a"
then any data you write will be appended on to the end of the file rather than replacing what might already be there:
Try running the code above multiple times and check inside the countdown.txt
file: you’ll see a new countdown is added each time you run the program
Writing CSV files
It can be really useful to organise the data you write to a file in a way that makes it easy to process in another program (such as a spreadsheet editor like Excel or LibreOffice Calc)
The easiest way to do this is to structure your data into a Comma Separated Values file (CSV). This just means your data is written to a text file but you write a comma ,
between every value and a new line \n
after every group of values.
The program below will ask the user to enter the scores for 5 people for a game and will write them to the file scores.csv
:
Below is an example of the CSV file scores.csv
(on the left) generated from the user input (on the right)
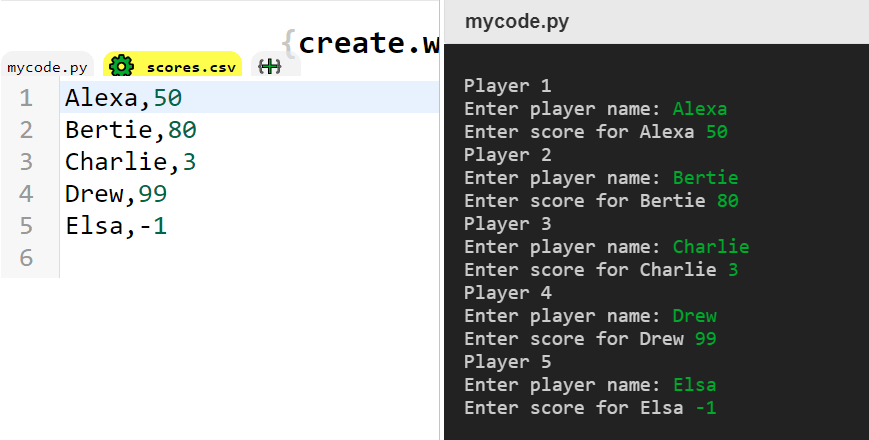
You can save the file you’re viewing (including .txt
and .csv
files) in create.withcode.uk by pressing the save/ share button (or Ctrl + Alt + S
)
You can then open the .csv
file in Excel or LibreOffice Calc and it will be displayed as a spreadsheet:
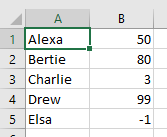
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.