The Theory:
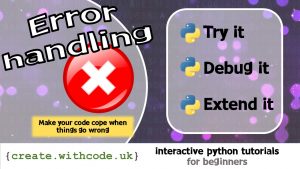
There are three types of error that could occur in your python code:
Syntax errors prevent your code from running at all because you’ve broken the rules (syntax) of the language. They’re the easiest to find and fix because you’ll always see an error message with a clue showing where the error is.
Runtime errors don’t prevent your program from starting (your code follows all the rules of the programming language) but they do cause your program to crash. They occur when your program tries to do something impossible (such as read a file that doesn’t exist)
Logic errors are the hardest to find and fix because you might not see an error message at all. They happen when your code does exactly what you told it to do, but you told it to do the wrong thing: there was a problem with the logic of your program.
Exceptions
When your program attempts to do something impossible, python will raise an exception which can cause your program to crash unless you’ve told it how to handle that exception.
Exception handling means you tell python which part of your code might go wrong and then you tell it how to cope if something does go wrong.
For example, if your code has to read data from a file, your code should be able to cope if the file doesn’t exist or if that file contains data that you weren’t expecting.
In python you do exception handling using try… except…
try
tells python that the indented block of code contains something that might potentially go wrong, but it should try to run it anyway.
except
tells python what to do instead of crashing if something goes wrong
For example, the code below asks you to enter the name of a file to display. If you type the name of a file that exists (e.g. hello.txt
) then it works, but if you type the name of a file that doesn’t exist, the program crashes due to a runtime error.
In order to make this code more robust, we can wrap the code that might crash with a try...except...
block:
This time, if you attempt to open a file that doesn’t exist you get a friendly error message and you can control how the program continues rather than it crashing completely.
Common exceptions
A runtime error can occur whenever the program tries to do something impossible. Common examples of this can be:
Divide by zero: dividing a number by zero is mathematically impossible (the result is infinity). You need to be careful using the divide operator (
/
) with a variable in case you accidentally divide by zero
Wrong data types: it’s often useful to from one data type to another (e.g. from string to integer) but sometimes it’s impossible. You need to be careful if you ever change the data type of data from the user in case they type in something you weren’t expecting them to.
Other common errors include:
- Reading from a file that doesn’t exist
- Writing a file that isn’t open in write mode
- Accessing data from a list outside of the size of that list
- Running out of memory
And many more. In each case, you can use try...except...
to detect and deal with the error before it causes your code to crash.
You can only use try...except...
to deal with runtime errors. They won’t detect logic errors and any syntax errors will prevent your code from running at all.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.