The Theory:
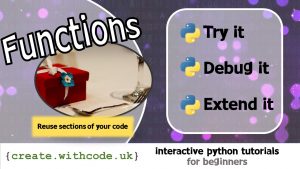
Just like a procedure, a function is a section of code that you can (re)use as many times as you like. Just like a procedure, functions have names (which should describe what they do).
Functions and procedures are both sub programs (they allow you to split code up into smaller parts).
A function returns a value. A procedure doesn’t.
The difference between a function and a procedure
The example below displays a random greeting (such as a Yorkshire “Ey up”).
Lines 3-6 define the function (tells python what to do)
Line 9 calls the function (tells python to actually do it)
Line 6 returns a value from the function to the line of code which called the function
How a function works
Defining a function means telling python what your function is going to do. The function definition starts with
def
followed by the name (identifier) then the code that makes the function work.
Lines 3-6 defineget_greeting
The identifier of a function is just the name of the function. It should describe what the procedure will do when you call it. The identifiers for functions have the same naming conventions (rules) as variables (use snake_case with underscores instead of spaces to describe what the procedure does)
The identifier of the function on line 4 isget_greeting
because the function chooses a random greetingCalling a function means telling python to actually run your function code. You call the function by writing the function name followed by round brackets
( )
(sometimes called parenthesis).
Line 9 callsget_greeting
(which makes lines 5-6 run)Returning a value means sending a value (that the function has calculated) back to the code which called the function. The return value is like the output of a function.
Line 6 chooses a random greeting and then returns it back to line 9 so it can be stored into the variablerandom_greeting
Built-in functions
Some functions are built in to python which means you can use them without having to tell python how they work.
The example below uses three built-in functions:
input
is a built-in function which asks a question and returns whatever the user types in. The return value forinput
is always a string (text)
Line 1 calls the functioninput
and stores the return value intoage_as_string
int
is a built-in function which converts a string (text) to an integer (whole number) .
Line 2 calls the functionint
and assigns the return value to the variableage_in_denary
bin
is a built-in function which converts a denary number (humans count in denary: 0-9) into binary (computers count in binary: 0-1)
Line 3 calls the functionbin
and assigns the return value to the variableage_in_binary
All of the above are built-in sub programs because procedures and functions are both types of sub program
Built in sub program
Advantages of using functions
Being able to create and use your own functions and procedures is a real break-through moment when you’re learning to program.
Using functions and procedures is an example of abstraction because you don’t need to know how the function works in order to call it
Abstraction means hiding unnecessary detail to focus on the most important information you need to solve a problem.
Making your own functions is an example of decomposition because you can break the program up into smaller parts
Decomposition means breaking a large problem down into smaller parts so that each part can be solved separately, making the whole problem easier to solve.
Call stack
Every time your program calls a sub program (procedure or function), it needs to know where to continue once the sub program has finished running.
Python does this by using a call stack.
A stack is a data structure where the last item you put in (store) is the first item you take out (read)
A data structure stores multiple values
Think of a call stack like a stack of paper. Each piece of paper contains a job that you need to complete. Whenever you add a new job to the stack, that takes over and you have to finish that job. Once you’re done, you remove that piece of paper from the stack and you carry on from where you left off.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.