Try it:
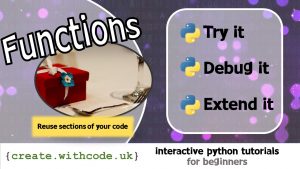
Below you’ll see some example python code which asks the user to enter a number and keeps asking until they do:
Press Ctrl + Enter
to run the code.
Here’s how the code works:
Lines 3-10 define a function called enter_number
. This tells python how to get the user to enter a number
Line 12 calls the function. This tells python to run the function code (make it actually happen)
Line 7 returns a value. This tells python to stop running the function and send a value back to line 12 where it is stored into the variable called value
Challenges:
Start by running the code.
- Call
enter_number
again and save the return value into a variable calledsecond_value
Line 12 calls
enter_number
and saves the return value into a variable calledvalue
so you can copy and adapt that line - Change the function to return a
float
(real / decimal number) instead of anint
eger (whole number)
Line 7 currently uses the
int
builtin function to convert the string you get from input into an integer. Use thefloat
builtin function instead - Change the function so that it only returns if the user enters a value over
0
You’ll need to use an
if
statement so that the function only returns if number is greater than 0
On the next page you’ll get some code with both syntax and logic errors
KPRIDE
KPRIDE stands for Keywords, Predict, Run, Investigate, Debug and Extend and it’s a way of helping you explore and understand python code. Click on the image below for a set of KPRIDE activities for this python skill.
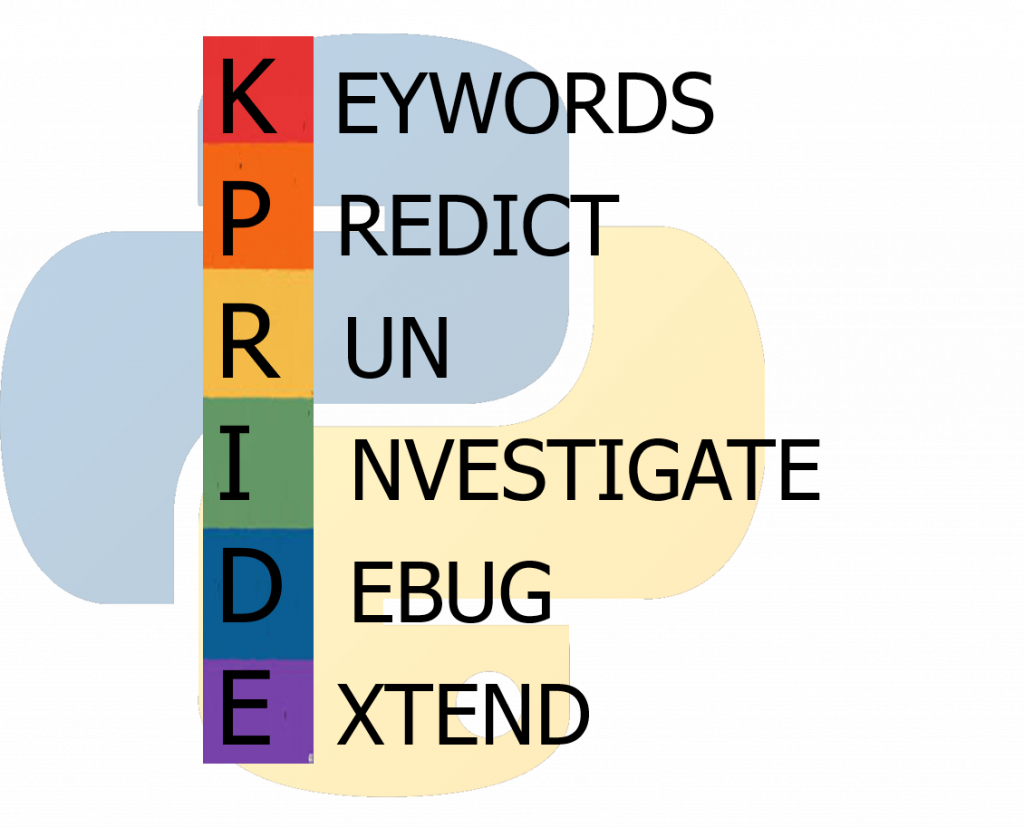
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.