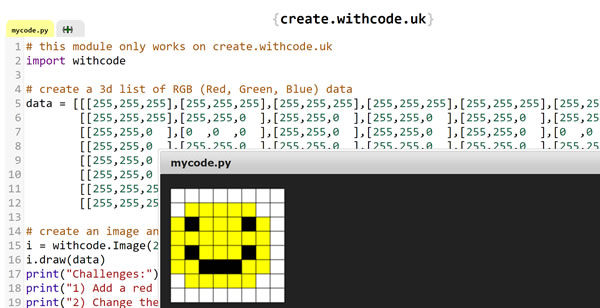
Welcome
This site is designed for teachers and students who want (or need!) to learn python programming.
This blog aims to have programming project ideas, links and resources that you can use at home or in the classroom but the main tool at the core of this site is create.withcode.uk which lets you write, run, debug and share python code on any device. Find out more here.
Python Tutorials
These beginners guides let you learn the basics of python then work through interactive challenges before generating a free PDF certificate to show off your progress
01: Python: Output
02: Python: Input
03: Python: Syntax errors
04: Python: Variables
05: Python: Naming Conventions
06: Python: Data Types
07: Python: Runtime errors
08: Sequence in Python
09: Selection in Python
10: Logic Errors in Python
11: Lists in Python
12: Iteration in Python
13: Writing data to a file in python
14: Reading data from a file in python
15: Error handling in python
16: Procedures in python
17: Functions in python
18: Parameters in python
19: Optimisation in python
The importance of comments in Python
Recent posts:
New season of live.withcode.uk: free python resources for GCSE Computer Science
by pddring | September 13, 2022 | Site news | 0 Comments
Adventures in Electronics 5: Tilt sensor car alarm
by pddring | January 3, 2025 | Adventures in Electronics | 0 Comments
Adventures in Electronics 4: Push button reaction timer
by pddring | December 30, 2024 | Adventures in Electronics | 0 Comments
Adventures in Electronics 3: 18B20 Temperature Sensor with motor
by pddring | December 26, 2024 | Adventures in Electronics | 0 Comments