This is a BBC micro:bit for beginners tutorial that shows you how to write some python code to turn a micro:bit into a sign you can stick to your bedroom door.
Python is a programming language that’s designed to let you write as little code as possible to make as much work as possible. Writing code on in python on a micro:bit is a great way to get your head around the essentials in python and hopefully have a lot of fun along the way too.
The micro:bit has an LED screen with just 5×5 pixels (dots). This isn’t much compared to the 2880×1800 pixels you might be used to if you’ve got a Macbook Pro but it’s big enough to display simple pictures and text one letter at a time.
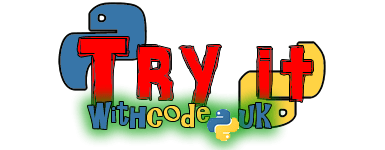
Display code on a BBC micro:bit
We’re going to be using the create.withcode.uk microbit simulator to write and test our code. To run the code you can press Ctrl + Enter or click on the run button in the bottom right of the code window.
If you want to test your code on an actual micro:bit, run the code in the simulator first then click on the Download Hex button. This’ll download a my_code.hex file that will run on your micro:bit when you drag it and drop it as though you were copying a file to USB memory stick.
There are two ways of displaying text to the micro:bit screen: display.scroll() and display.show(). What’s the difference? Which do you prefer?
Let’s go through each of the lines in turn:
import microbit microbit.display.scroll("Hello") microbit.display.show("World")
Line 1 tells python to import the microbit module. That means that python loads some extra code which tells it how to control a microbit. Lines like this, where you’re importing a module, usually go at the top of your code.
import microbit microbit.display.scroll("Hello") microbit.display.show("World")
Inside the microbit module there’s another module called display. Inside display is a procedure called scroll. The round brackets after scroll tell python to call (run) that procedure.
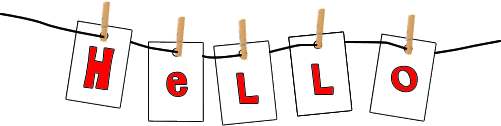
A “string” of characters
Notice the text in the brackets is surrounded by quotation marks: we call this a string because it joins the characters together as though they were threaded on a string like a washing line.
The scroll procedure scrolls the text from right to left, moving it one pixel at a time.
import microbit microbit.display.scroll("Hello") microbit.display.show("World")
Line 3 is similar to line 2 but instead of scrolling the text pixel by pixel, we show one character at at time.
You might notice in some tutorials that instead of import microbit you see from microbit import *
Both of these tell python to load code from the microbit module, but the second means that you don’t have to write microbit. every time you use the microbit module:
from microbit import * display.scroll("Hello") display.show("World")
import microbit microbit.display.scroll("Hello") microbit.display.show("World")
Both of the two programs above will do the same thing when they run. The top one is easier to write but some people prefer the bottom one to save confusion later in the development process.