The Theory:
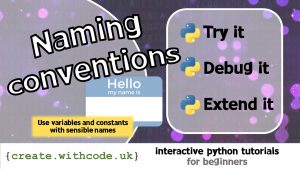
Python naming conventions: Use variables and constants with sensible names
We’ve already learnt that variables are like boxes that store some useful stuff.
Imagine moving house, then sorting through 100s of boxes to try to find something important. If your boxes are clearly labelled, it makes finding what you need so much easier and less frustrating.
Also, you might need some boxes more than others: some might always keep the same stuff in all the time. You might need other boxes all the time.
Variables are like boxes that you add stuff to or take stuff out of all the time. Constants are like boxes that always store the same stuff.
Constants are just like variables in that they also have a name and a value.
The only difference is that you set the value of a constant once and then it always stays the same (constant)
When you use variables and constants in python, you’re supposed to follow a set of rules. These rules are called naming conventions.
Your code will still run if you break these rules, but they make your code much easier for you and other people to understand, which means you’re less likely to make mistakes later on.
You can find out more about the python naming conventions here, but here’s a summary of the main points:
- Variable names should be in lowercase letters with words separated by underscores (
_
):
e.g.
my_name = "Betty"
is good butMyName = "Betty"
is notIn python, you’ll get an error message if you try to make a variable name with a space in it - Constant names should be in uppercase letter with words separated by underscores (
_
):
e.g
max_score = 10
is OK butMAX_SCORE = 10
is better if a game’s maximum score doesn’t change at all while the program runs.Constants are useful for giving certain settings, numbers or data a name. This makes your code easier to read and update.
- Both variable names and constant names should describe the data that they store:
e.g.
score = 0
is good butmy_little_variable = 0
is pretty unhelpful.
Occasionally, you might use really short variables likei = 0
for counting but it helps if you make your variable names really clear
We’re going to learn how to write python code that follows naming conventions so that it’s easy to read and maintain. Maintaining code means going back and improving or fixing it in the future, often when you’ve completely forgotten what parts of it do.
Key point: Use
variables_like_this
to store data that might change whilst your program runs.Use
CONSTANTS_LIKE_THIS
to store data that you might use lots, but only ever set once, often at the start of the program.
On the next page you’ll get some code examples that you can try out for yourself.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Trackbacks/Pingbacks