The Theory:
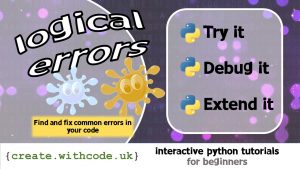
There are three types of errors you’ll come across with your code: Syntax errors, run time errors and logical errors.
Syntax errors happen when you break the rules of the language like accidentally missing out a
"
or)
. Syntax errors stop your code from running at all.Runtime errors happen when your code tries to do something that’s impossible like trying to convert the text
"potato"
into an integer number data type. Runtime errors make your program crash whilst it’s trying to do the thing that’s impossible.Logic errors happen when your code does what you told it to do, but not what you wanted it to do, like adding two numbers instead of subtracting them. Logic errors might not crash your program but will cause it to do the wrong thing.
The easiest of the three types of errors to find are syntax errors because you get an error message appearing as soon as you try to run your code.
Finding and fixing runtime errors is easier than debugging logic errors because you will at least get an error message that usually tells you which line of code that is broken.
Logic errors are harder to find because you might never see an error message and your program might never crash.
In order to find logic errors, you have to test each part of your code to make sure that it behaves as you want it to and gives the results you’re expecting.
If you make a logic error it means that you’ve made a mistake when converting your algorithm into code.
Algorithm means step by step instructions to solve a problem
There’s no way to avoid ever making logic errors, but these tips should help you plan to avoid making logic errors and test thoroughly to detect any pesky logic errors you missed whilst programming:
- Plan before you program
If your code has to make any decisions or process any data it helps to write down what you want it to do.
You could do this as a diagram (like a flow chart) or by writing out the algorithm using pseudocode or a written description of what it should do
- Comment your code
Describing what your code should do makes it much easier to spot when your code actually does something else.
- Test with valid data
When testing your code, you’ll need to put in test data to see what each part of the program does when the user types in normal, sensible values.
For example, if your program asked for a number between
1
and10
, you should test it works properly with a number like3
- Test with boundary data
You should test each part of your program with values that are right on the edge of what the program is expecting.
For example, if your program asked for a number between
1
and10
, you should test it with both1
and10
- Test with invalid data
You should make sure your program can cope with values outside of the range it would normally expect. For example, if your program asked for a number between 1 and 10, you should test it with
0
and43
- Test with erroneous data
You should make sure your program can cope with values that are a different data type to the one that it’s expecting.
For example, if your program asked for a number between 1 and 10, you should test it with bob
If you’re testing each of these scenarios you’ll hopefully catch all of the logic errors, but, more importantly, if you’re thinking of all of these scenarios, you’re much more likely to avoid writing those logic errors in the first place.
On the next page you’ll get some code examples that you can try out for yourself.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Shocking website!! I could not get the output without changing the browser which is not what a website should do!
Shocking feedback!! Please could you let me know what browser you were using and what problem you had so I can improve the shocking website painstakingly put together for free in my spare time. It’s a long way from perfect. Alternatives are available.
Hiya, great website, the pound sign(£) creates a unicode error and the code will not run. If you delete the £’s it runs fine. I have tried this in Edge and Chrome.
Hello,
Thanks so much for getting in touch and reporting that bug. It should now be fixed – all strings should support unicode characters. Sorry about that!
All the best 🙂