The Theory:
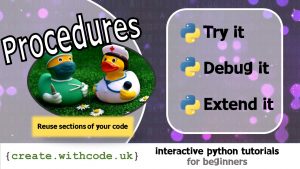
A procedure is a section of code that you can (re)use as many times as you like. Procedures have names (which should describe what they do).
The example below draws 5 squares in random positions. It creates and uses two procedures draw_square
and teleport
Defining a procedure means telling python what your procedure is going to do. The procedure definition starts with
def
followed by the name (identifier) then the code that makes the procedure work.
Lines 6-9 definedraw_square
and lines 12-17 defineteleport
The identifier of a procedure is just the name of the procedure. It should describe what the procedure will do when you call it. The identifiers for procedures have the same naming conventions (rules) as variables (use snake_case with underscores instead of spaces to describe what the procedure does)
Calling a procedure means telling python to actually run your procedure code. You call the procedure by writing the procedure name followed by round brackets
( )
(sometimes called parenthesis).
Line 21 callsteleport
(which makes lines 13-17 run) and line 22 callsdraw_square
(which makes lines 7-9 run)
Why use procedures?
If you find yourself writing out the same code more than once then it’s probably a good idea to use a procedure or function instead.
The code below does exactly the same thing as the code above (draws 5 squares in random positions) but without using procedures or for loops:
Whilst both examples do exactly the same thing, having lots of repeated lines of code is not as good for the following reasons:
Efficiency: writing out the same lines of code multiple times means it takes longer for you to write out your program
Maintenance: having the same lines of code multiple times means that if you need to change something, you’ll have to make that change to multiple lines of code
Debugging: having the same lines of code multiple times means you’re more likely to make mistakes and finding those mistakes is harder
If we used for loops but avoided using procedures we can make the code even shorter than the example at the top of the page:
This code might be shorted but it’s less readable and less reusable
Readability means how easy it is for other programmers to understand what your code does. Making your code easy to read means you’re less likely to make mistakes and your code will be easier to debug.
Using procedures improves code readability cause the names of procedures (e.g.
draw_square
) should describe what that section of code actually do (e.g. draw a square)
Reusability means how easy it is for you or other programmers to reuse sections of your code in other projects or programs.
Using procedures makes it easier to reuse sections of code because each procedure is self-contained with its own set of local variables so you should be able to copy and paste a procedure from one python file to use it in another project.
Local and global variables
When you make a variable on a line of code that has no spaces or tabs at the start then it’s a global variable. This means that it can be accessed anywhere else in that python program.
When you make a variable inside a procedure (or function) it is a local variable. This means that it can only be accessed within the same procedure (or function).
The scope of a variable means the part of the code where that variable can be accessed (variables either have global scope or local scope depending on where they are first used in a program)
Going back to the example that uses procedures to draw 5 random squares, the code below has both global and local variables:
Variable | Scope | Explanation |
t | global variable | Defined outside any procedures or functions on line 3, t can be accessed anywhere in the code (it’s used in both draw_square and teleport |
x | local variable | Defined inside the procedure teleport on line 14, you’d get an error message if you tried to access x anywhere from any other part of code other than within the teleport procedure |
i | global and local variables | There are two different variables with the name i . Line 7 creates a local variable inside the draw_square procedure. This is used to count to 4 (to draw each side of the square).There is a separate global variable defined on line 20 with the same identifier ( i ) which is used to count to 5 (to draw 5 different squares) Both variables can have different values because they exist in different scopes (one is local to draw_square and the other is global) |
Having different variables in different scopes can be confusing, especially if there’s more than one variable with the same name in different scopes (e.g. i
in the example above).
The advantage of variable scope is that each procedure can have its own set of local variables which don’t rely on or interfere with any other part of the code. This can help make a procedure easier to debug and easier to re-use in another project.
It’s considered bad practice to use global variables to store all your data because it’s much harder to keep track of which parts of your code change or rely on each variable.
Using local variables is better because they are contained inside the procedures / functions and so won’t interfere with or overwrite data needed by other parts of the code that might accidentally use the same variable name.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.
Hi there,
Absolutely love your resources on programming and started using them today. Just wondering if there are solutions for the de-bugs on the programs here that pupils are supposed to de-bug. It will help me a lot so I can compare their answers with it.
Hello,
Thanks so much for your message. I haven’t written up the answers yet – I’m trying go get through the full set of 20 activities then the next job will be to publish a student workbook and set of answers. Sorry not to help yet. Hope you’re well