The Theory:
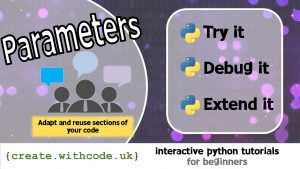
Parameters let you pass data into a function or procedure.
Functions and procedures are both sub programs
A function returns a value. A procedure doesn’t.
A return value is the output from a function
Parameters are the inputs to functions and procedures
Parameters are treated like local variables within a sub program.
The example below draws two squares: a large square with a smaller one inside.
Lines 5-8 defines the procedure called
draw_square
(tells python how to draw a square). It’s a procedure because it doesn’t return a valueThe brackets on line 5 tell python that there’s one parameter called
size
Line 7 uses the parameter
size
as though it’s a local variableLine 10 calls
draw_square
, passing100
to the procedure as the value forsize
Line 11 calls
How parameters workdraw_square
again with a different value for thesize
parameter
Parameters, global and local variables
A local variable is a variable that is defined inside a sub program (function or procedure). It can only be read or set within the scope of that sub program.
A global variable is a variable that is defined outside of a sub program which means it can be read or set anywhere in the program.
A parameter behaves in a similar way to a local variable in that it can be accessed from within a sub program but the big advantage is that you can set its value from outside the sub program, when that sub program is called.
Below is the same program written in different ways. Both programs can say hello in two languages, French and English. The first example uses a global variable to decide which language to greet the user in:
The example below has been adapted to pass the language as a parameter rather than a global variable:
Passing data as parameters is considered better practice than using lots of global variables because any part of a large program could change a global variable and accidentally break another part. Using parameters makes code more modular, which means it’s easier to adapt, extend, debug and re-use in other projects in the future
You can pass data as literal values, expressions or variables into subprograms using parameters
A literal value is something like
"hello"
,0
orTrue
(a simple value rather than a variable or expression)A variable is able to store a literal value (e.g.
hungry = True
)
When you pass a variable as a parameter, a copy of the value stored in that variable gets sent to the sub programAn expression is a combination of variables, operators (like add and subtract), and function calls (e.g.
Expressions, variables and valueslen(message) - 1
).
When you pass an expression as a parameter, the expression is first evaluated (which means calculated or processed) into a value before being passed to the sub program
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.