The Theory:
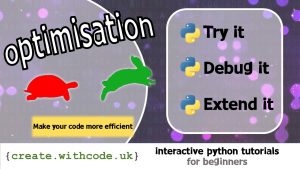
There are three ways to optimise your code:
Optimising for speed means making your code run as quickly as possible. This means the user doesn’t have to wait long for the program to finish
Optimising for memory usage means your program won’t need as much RAM (memory) to store all of the data whilst it runs. This means your program will work on computers with less RAM or will allow users to have more programs open at the same time as your program.
Optimising for size means reducing the number of lines of code you need to write. This means that your program will take up less space on disk.
A good general principle is D.R.Y:
Don’t
Repeat
Yourself
If you can avoid running lines of code more times than necessary then your program will run as quickly as possible.
If you can avoid storing data in multiple places then your program will use less memory
If you can avoid having lots of lines of code which are similar to other parts of a program then your code will be as short as possible.
Sometimes it is a balancing act between different priorities: to make your program run faster you might need to make it use more memory. Or making your code use less memory might make it slower.
Example: Reducing the number of lines of code
Reducing the number of lines of code in your program is usually a good thing as it makes your code easier to maintain as well as taking up less space when you save your code. But if you reduce the lines of code too much (or strip out all the comments to make it shorter) it makes it harder to understand and maintain in the future.
The code below draws a 12 point star using Python’s turtle graphics
Lines 5-6 are repeated 12 times. This is inefficient and can be optimised to make the code shorter.
Shorter code means it’s easier to find and fix any mistakes and the code takes up less space when you save it.
The code below does exactly the same thing but uses a for loop to optimise the code to avoid repeated lines of code:
Example: Improving speed
Understanding a bit about computer hardware helps you understand how to get the most performance out of your code.
Reading and writing to memory (e.g. reading or setting the value of a variable in RAM) is usually very fast
Using functions or performing calculations with data is slower than reading individual values from memory
Reading and writing to a hard disk (e.g. loading data from a file on the HDD) is much slower
Reading and writing to a network (e.g. downloading data from the Internet) is even slower
If you want to speed up your program, you want to avoid repeating any slow operations.
The following code has a text file which stores some random user details. The python code asks the user to type in a user ID (from 1 to 100) and then attempts to look up the details of that user:
The code works, but every time the user tries to search for a user, the program will load the whole user database from disk. This is much slower than it needs to be.
The following code does the same thing (lets the user search for someone from the file) but this time it loads the file into memory once so that that it doesn’t have to be read from the slow hard disk every time you search for a user:
You will notice that this code is longer (there are more lines of code) but it will run much faster because the file doesn’t need to be loaded from disk each time you search for a user. The data is loaded from disk once and then stored in a list (in memory, which is faster than a hard disk or solid state drive).
Optimisation Tips
Optimising your code is an art: this article is only designed to give an introduction and quick overview, but here are some quick tips:
Using local variables instead of global variables can reduce the amount of memory your program uses
Wherever you see a section of code that does something similar more than once, you should use a for or while loop instead so that your program is shorter, easier to read, easier to maintain and takes up less space when you save your code
Wherever you see a for or while loop to repeat a section of code, see if you can take any code that doesn’t need to be repeated out of the loop so that your code run more quickly
Choose your algorithm wisely, especially if you are searching for or sorting data. Some algorithms are better at using less memory and some are better at being faster.
Choose your data structures carefully. These tutorials focus on individual variables and lists but other data structures such as dictionaries and tuples might allow you to make your code much more efficient.
In real life, you can use a dictionary to look up the definition of almost any word. In Python, you can use a key to look up a value
Dictionary: data structure that allows you to link pairs of keys and values so that you can use a key to look up its value
Key: the data that you use to look up a value from a dictionary (e.g. unique ID number or username)
Value: the data that you get when you use a key to look something up in a dictionary
If you store your data in a list, it could take a long time for your program to find that data as it might have to search through every value in that list to find what you’re looking for.
If you store your data in a dictionary, your program can find it almost instantly because you link values to a key, which can be used like the index of a list to find the data you need, even if you don’t know the order or position of the data you’re trying to find in the dictionary.
The code below loads the same user details as the previous example but this time saves them into a dictionary rather than a list.
Line 3 creates a new dictionary (notice the { }
brackets instead of [ ]
for a list
Line 17 adds user details to the dictionary. For each user, the key is the user’s id
and the value associated with that key is a list containing all that user’s details: [id, first_name, last_name, ip_address]
Line 28 looks up a value from the dictionary, using the id_to_search_for
as a key and getting a list of that user’s details
Using inefficient data structures like lists won’t be a problem for small amounts of data like this, but if you had millions of users to work through, you could optimise your program to run much, much faster if it didn’t have to trawl through every single user account in a list but could find it instantly from a dictionary instead. However, a dictionary would use a little more memory than a list.
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.