Try it:
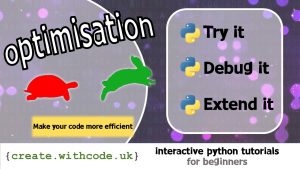
Below you’ll see some python code which will draw five different 5 pointed stars. It’s horrible inefficient and needs to be optimised to do the same thing in fewer lines.
Press Ctrl + Enter
to run the code.
Here’s how the code works:
Lines 1-5 set up the python turtle graphics module for drawing the stars.
Lines 6-16 draws a single 5 pointed star. These lines are repeated almost exactly to draw the other 4 stars.
Challenges:
Start by running the code.
- Make a procedure called
draw_star
which lets you draw a star at any position
You will need to use
def
to define a procedure. The procedure will need two parameters to set thex
andy
coordinates of the star. - Use a
for
loop to draw each of the 5 points of each star
The
forwards(20)
andright(144)
instructions are repeated 5 times for each star. Put these inside a for loop to reduce the number of lines of code required to draw the star - Add a third parameter to your
draw_star
procedure so that you can change the size of each star
At the moment each star is the same size (20 pixels). Change the procedure definition to accept three parameters (
x
,y
andsize
)
On the next page you’ll get some code with both syntax and logic errors
KPRIDE
KPRIDE stands for Keywords, Predict, Run, Investigate, Debug and Extend and it’s a way of helping you explore and understand python code. Click on the image below for a set of KPRIDE activities for this python skill.
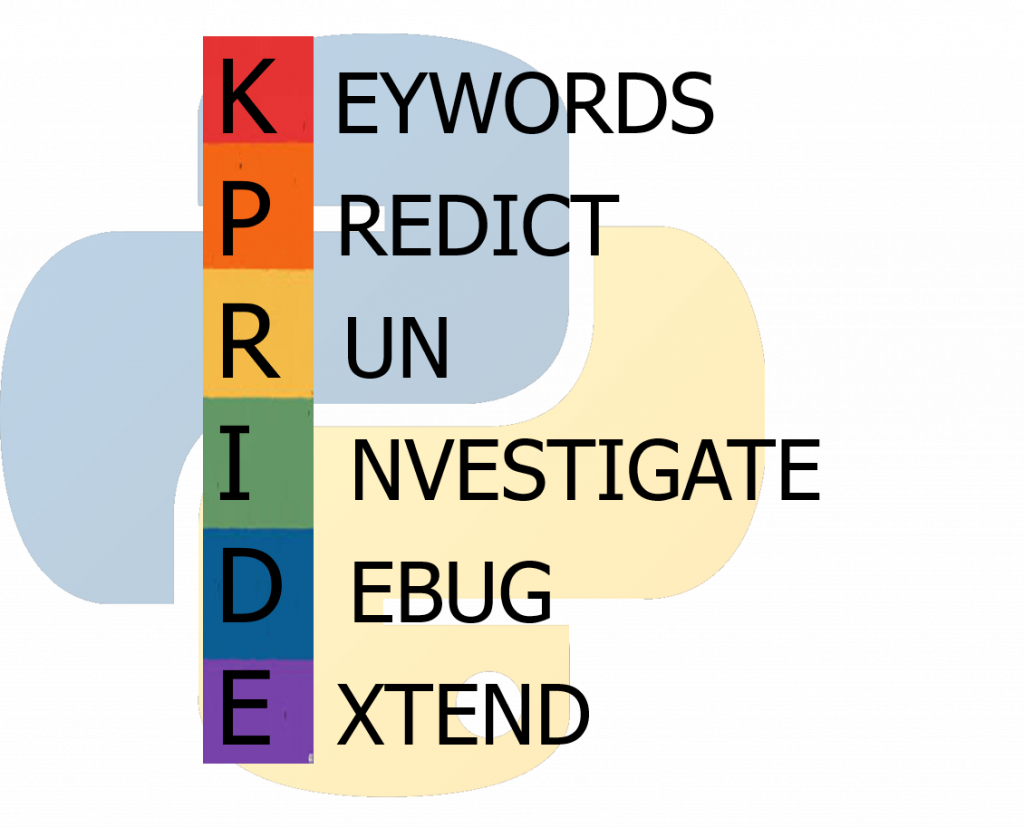
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.