Stage 5: Show those pipes
So far, all we’ve got is a bird that flaps up and falls down. Not much of a game. As soon as we add a pipe that we’ve got to dodge, it starts to get much more fun.
The pipes will eventually scroll across the screen from right to left. They’re going to have a gap of 2 LEDs for the bird to try to squeeze between. We want them to appear in a random position so that sometimes you’ve got to flap to the top of the screen, sometimes to the bottom and sometimes in the middle.
- Add the line import random near the top, under the line that says from microbit import *
This imports the random module, which lets us choose a random number. We need a random number so that the pipes don’t always show in the same place. - Above the line that starts the while True: loop, add in the following lines:
# Flappy bird Stage 5: Dodge those pipes # https://blog.withcode.uk/2016/05/flappy-bird-microbit-python-tutorial-for-beginners from microbit import * import random display.scroll("Get ready...") y = 50 speed = 0 score = 0 # Make an image that represents a pipe to dodge def make_pipe(): i = Image("00003:00003:00003:00003:00003") gap = random.randint(0,3) # random wall position i.set_pixel(4, gap, 0) # blast a hole in the pipe i.set_pixel(4, gap+1, 0) return i # create first pipe i = make_pipe() # Game loop while True: # show pipe display.show(i) # flap if button a was pressed if button_a.was_pressed(): speed = -8 # show score if button b was pressed if button_b.was_pressed(): display.scroll("Score:" + str(score)) # accelerate down to terminal velocity speed += 1 if speed > 2: speed = 2 # move bird, but not off the edge y += speed if y > 99: y = 99 if y < 0: y = 0 # draw bird led_y = int(y / 20) display.set_pixel(1, led_y, 9) # wait 20ms sleep(20)
Lines 13 to 18 define a new function called make_pipe . Line 21 uses this function to create a new image of a pipe called i .
i = Image(“00003:00003:00003:00003:00003”) creates a new image for a micro:bit called i . This is a local variable which means it only exists in the function it’s defined in. That’s a confusing way of saying that we can’t access, use or change this local variable called i anywhere other than inside make_pipe (lines 14-18).
Images on micro:bit
The numbers represent the brightness of the LEDS and the colons (:) represent a new line. So this image is a dim line from top to bottom on the far right of the screen.
Line 15 chooses a random position for a hole in this line:Â gap = random.randint(0,3)Â and lines 16 and 17 replace the pixels at that position and the pixel below it with zero brightness to turn the LEDs off.
- Next, we need to display the pipe image. We’ll do this instead of clearing the display. Find the line that said display.clear()Â and replace it with display.show(i)
We don’t need to clear the display any more, because our image of a pipe not only tells the micro:bit to display the pipe but also to switch off all of the other LEDs. We could keep display.clear() and put display.show(i) underneath but when coding games you always try to make your code run as efficiently as possible by removing anything that takes up unnecessary processing time and resources.
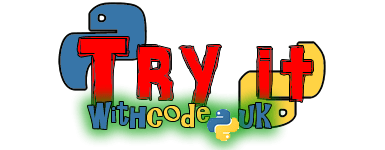
Try it so far
We’re almost there now. Try out the code we’ve got so far below and make a list of the things that you think we still need to do to finish our game:
Your code produces a “TypeError” on line 71.
Hi Darryl,
Thanks – you’re right – sorry about that. I made a mistake when making micro:bit python simulator for the Image.shift_left() function – it ignored the parameter, which your comment has helped me fix: cheers!
I’ve changed line 71 from
i = i.shift_left()
toi = i.shift_left(1)
which should fix the problem when you test it on a micro:bit.Great. Perfectly explained….
GREAT!!!!!!!!!!!!!!!
amazing
great ! thanks
cool 🙂
this is totally awsome \_(*-*)_/
I have a name error on line 22. It says name not defined.
Thanks for getting in touch, sorry about the error. Please could you post a link to your code or let me know which example you’re getting the error with?
I’ve got the code https://create.withcode.uk/python/58B
It is at the section of
def make_pipe():
i = Image("00003:00003:00003:00003:00003")
gap = random.randint(0,3)
i.set_pixel(4, gap, 0)
i.set_pixel(4, gap+1, 0)
return i
The micro bit shows 'name error' ' name not defined'
Hope this helps
Thanks
I’ve edited your comment to replace the code with a link if that’s ok? – posting python directly on here gets rid of indentation and swaps some characters like quotation marks. I can’t seem to get the same error as you on the simulator or a micro:bit. Please could you check the link and see if it works for you or if the code matches yours?
I’ve tested the code you post on micro bit and it seems like it’s still having the same error message.
Line 17 ‘name error’ name ‘random’ is not defined.
Sorry my misunderstanding with your comment, i thought you fixed the issue. Yes the code matches my original code that I’ve got.
Sorry-that’s very frustrating. It runs on a microbit without an error for me. Are you getting the hex file from create.withcode.uk when you run the simulator or are you using a different editor? Could you try clearing your browser cache in case you’ve got an old version of the runtime stored. You’re the second person to say there’s a problem with the random module so I’d like to track down what the issue is, but I can’t replicate the problem on any browser. Thanks for your patience and help.
Hi, I tried clearing the cache and the same error occurs again. I’m using https://python.microbit.org/v/1 to edit my coding. Normally I would save the file from as a .py file and drag the file into the Micro:Bit folder.
Hope those information helps
Thanks – that’s helpful.
The micro:bit can’t run .py files directly: micro:bits need to be flashed with .hex files which contain both your python code and the micropython runtime which tell the micro:bit how to interpret your code. In the https://python.microbit.org/v/1 editor you’re using, you need to press the download button to create a hex file. That’s the one you then drag into the micro:bit folder. In create.withcode.uk, press ctrl + enter to run your code and you’ll see a link above the simulator that will let you download the hex file.
Hope that helps.
Hello, your code seems great, i some what tried to base my game off yours. Mine is a car dodging game (the car will be positioned in the bottom-middle) and there will be cars coming down from the top. The problem is that, i have made 2 opposition cars but only one of them is displaying and one of them is not, please let me know why?? (I haven’t done everything yet, such as dying and duplicating the opposition car code, because i got stuck with this problem). I feel like it has something to do with display.show??
Hello,
Thanks for getting in touch. Nice idea for a game. The trick is to combine two images using + rather than and.
Change line 55 to display.show(b+c) and you’ll show both images merged together. You can find out more here: https://microbit-micropython.readthedocs.io/en/latest/image.html
Hope that helps. Do share the final version!
Sorry for disturbing you again, and thank you so much for the quick reply (and it worked, yay!!) . This time i have added in all of the 5 cars and the death situation, but the problem this time is that all of the 5 cars come down at the same time and therefore, the car which the player gets to control cannot escape. I really do not know how to change this but i feel like i have to use random. This is because i feel like each car should come down at a random time when it is at the top therefore, leaving slight gaps for the players car to escape. But i tried to use random.randit but it doesnt work with time. Why doesnt random work with time??
Sorry for disturbing you again, and thank you so much for the quick reply (and it worked, yay!!) . This time i have added in all of the 5 cars and the death situation, but the problem this time is that all of the 5 cars come down at the same time and therefore, the car which the player gets to control cannot escape. I really do not know how to change this but i feel like i have to use random. This is because i feel like each car should come down at a random time when it is at the top therefore, leaving slight gaps for the players car to escape. But i tried to use random.randit but it doesnt work with time. Why doesnt random work with time??
it still aint working
Would you be able to describe the problem you’re having and I’ll do my best to help fix it 🙂