Step 2: Display the bird
In case you’ve been living on another planet for the last few years and have never played flappy bird: the bird itself doesn’t move left of right – only up and down.
We need to keep track of where it is vertically (the y axis). There are only 5 rows of LEDs on the micro:bit screen but in order to make the bird flap up and down more realistically, we’re going to pretend that there are 100 different positions the bird can be on the y axis and then scale this down to display it on the LED grid:
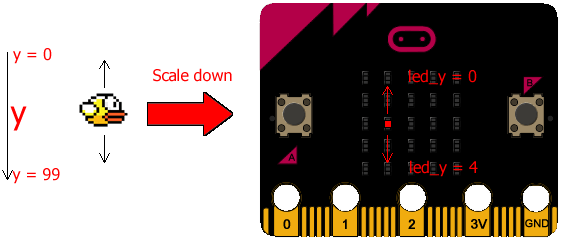
Scaling down y coordinates to fit on the 5×5 LED screen
- Write y = 50 at the bottom of your code.
This makes a new variable called y.
A variable stores some data that might change when the program runs. This variable stores the y coordinate (from 0: top to 99:bottom) and we set it to 50 at the start of the game.
- Add the line led_y = int(y / 20) to the bottom of your code.
This makes a new variable called led_y which stores a number from 0 – 4, telling us which row of the LED screen to light up.
y / 20 divides the value stored in the y variable by 20 so that we can scale down the bird coordinate to fit on the LED screen.
The int() function rounds the result of y / 20 down to a whole number (an integer is a whole number with no decimal places)
- Add the line display.set_pixel(1, led_y, 9) to the bottom of your code.
This turns on one pixel which will represent our bird.
There are three parameters for set_pixel: x, y and brightness. We’re setting the x coordinate to 1. The x coordinate for the first column is 0, so our bird will be on the second column.
We’re setting the y coordinate in set_pixel to led_y. led_y will be 2 (y was 50 and we divided that by 20 and rounded down). This means the bird will be on the third row down (the first row is row 0)
The 9 as the last parameter in set_pixel is the brightness. This can be any number from 0 to 9 with 0 meaning completely off and 9 meaning as bright as it’ll go. We’re going to have a bright LED for the bird and dim LEDs for the pipes that you’ve got to try to avoid.
- Your code should now look like this. The new lines are highlighted.
from microbit import * display.scroll("Get ready...") y = 50 led_y = int(y / 20) display.set_pixel(1, led_y, 9)
The code below contains some common mistakes. See if you can find and fix them all.
Your code produces a “TypeError” on line 71.
Hi Darryl,
Thanks – you’re right – sorry about that. I made a mistake when making micro:bit python simulator for the Image.shift_left() function – it ignored the parameter, which your comment has helped me fix: cheers!
I’ve changed line 71 from
i = i.shift_left()
toi = i.shift_left(1)
which should fix the problem when you test it on a micro:bit.Great. Perfectly explained….
GREAT!!!!!!!!!!!!!!!
amazing
great ! thanks
cool 🙂
this is totally awsome \_(*-*)_/
I have a name error on line 22. It says name not defined.
Thanks for getting in touch, sorry about the error. Please could you post a link to your code or let me know which example you’re getting the error with?
I’ve got the code https://create.withcode.uk/python/58B
It is at the section of
def make_pipe():
i = Image("00003:00003:00003:00003:00003")
gap = random.randint(0,3)
i.set_pixel(4, gap, 0)
i.set_pixel(4, gap+1, 0)
return i
The micro bit shows 'name error' ' name not defined'
Hope this helps
Thanks
I’ve edited your comment to replace the code with a link if that’s ok? – posting python directly on here gets rid of indentation and swaps some characters like quotation marks. I can’t seem to get the same error as you on the simulator or a micro:bit. Please could you check the link and see if it works for you or if the code matches yours?
I’ve tested the code you post on micro bit and it seems like it’s still having the same error message.
Line 17 ‘name error’ name ‘random’ is not defined.
Sorry my misunderstanding with your comment, i thought you fixed the issue. Yes the code matches my original code that I’ve got.
Sorry-that’s very frustrating. It runs on a microbit without an error for me. Are you getting the hex file from create.withcode.uk when you run the simulator or are you using a different editor? Could you try clearing your browser cache in case you’ve got an old version of the runtime stored. You’re the second person to say there’s a problem with the random module so I’d like to track down what the issue is, but I can’t replicate the problem on any browser. Thanks for your patience and help.
Hi, I tried clearing the cache and the same error occurs again. I’m using https://python.microbit.org/v/1 to edit my coding. Normally I would save the file from as a .py file and drag the file into the Micro:Bit folder.
Hope those information helps
Thanks – that’s helpful.
The micro:bit can’t run .py files directly: micro:bits need to be flashed with .hex files which contain both your python code and the micropython runtime which tell the micro:bit how to interpret your code. In the https://python.microbit.org/v/1 editor you’re using, you need to press the download button to create a hex file. That’s the one you then drag into the micro:bit folder. In create.withcode.uk, press ctrl + enter to run your code and you’ll see a link above the simulator that will let you download the hex file.
Hope that helps.
Hello, your code seems great, i some what tried to base my game off yours. Mine is a car dodging game (the car will be positioned in the bottom-middle) and there will be cars coming down from the top. The problem is that, i have made 2 opposition cars but only one of them is displaying and one of them is not, please let me know why?? (I haven’t done everything yet, such as dying and duplicating the opposition car code, because i got stuck with this problem). I feel like it has something to do with display.show??
Hello,
Thanks for getting in touch. Nice idea for a game. The trick is to combine two images using + rather than and.
Change line 55 to display.show(b+c) and you’ll show both images merged together. You can find out more here: https://microbit-micropython.readthedocs.io/en/latest/image.html
Hope that helps. Do share the final version!
Sorry for disturbing you again, and thank you so much for the quick reply (and it worked, yay!!) . This time i have added in all of the 5 cars and the death situation, but the problem this time is that all of the 5 cars come down at the same time and therefore, the car which the player gets to control cannot escape. I really do not know how to change this but i feel like i have to use random. This is because i feel like each car should come down at a random time when it is at the top therefore, leaving slight gaps for the players car to escape. But i tried to use random.randit but it doesnt work with time. Why doesnt random work with time??
Sorry for disturbing you again, and thank you so much for the quick reply (and it worked, yay!!) . This time i have added in all of the 5 cars and the death situation, but the problem this time is that all of the 5 cars come down at the same time and therefore, the car which the player gets to control cannot escape. I really do not know how to change this but i feel like i have to use random. This is because i feel like each car should come down at a random time when it is at the top therefore, leaving slight gaps for the players car to escape. But i tried to use random.randit but it doesnt work with time. Why doesnt random work with time??
it still aint working
Would you be able to describe the problem you’re having and I’ll do my best to help fix it 🙂