In this stage we’re going to make it so that you can shake the micro:bit to stoke up the fire when it burns down too low.
- Add the final bits of code that are highlighted:
from microbit import * import random # create an empty image i = Image("00000:"*5) # start with the fire at medium intensity intensity = 0.5 # keep looping while True: # show the image and wait display.show(i) sleep(100) # shake the micro:bit to stoke the fire if accelerometer.was_gesture("shake"): intensity = 1 # shift the image up and fade it slightly i = i.shift_up(1) * intensity # let the fire burn down a little (reduce the intensity) intensity *= 0.98 # choose random brightness for bottom row of fire for x in range(5): i.set_pixel(x, 4, random.randint(0,9))
Line 17 checks to see if the micro:bit has been shaken. If it has, line 18 sets the value stored in the variable called intensity to 1.0. This is called conditional code or selection because your code is making a choice / selection based on a condition (in this case, has it been shaken)
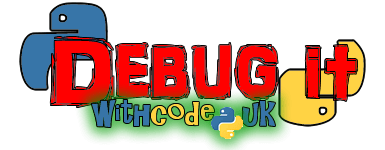
Find and fix the errors
Below is some code that has been deliberately sabotaged with three errors:
A syntax error is stopping the code from running at all.
A runtime error is making the program crash after it’s started to run
A logical error is making the program behave differently to what we’d like.
- See if you can find and fix the errors in the code below:
The final page gives you some ideas for how to extend and improve this project