The Theory:
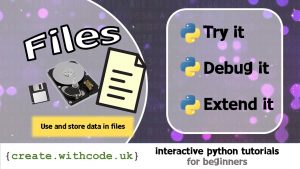
Computers have two types of memory to store data: primary storage and secondary storage.
Primary storage (e.g. Random Access Memory) is very fast but only stores data whilst the power is switched on.
Secondary storage (e.g. Hard Disk Drive) is slower but data is stored even when the computer is switched off.
Reading from files
When you load data from a file you are usually reading data from secondary storage and putting it into primary storage, which is much faster but can’t store as much data.
Loading data allows you to process it (make calculations using the data).
Reading data from a file in python is really easy using the open
and read
functions:
The challenging part is usually knowing how to process and store the data that you read from the file.
If you want to read the whole contents of a file in one go, you can use use the read()
function. The example below reads the 10 most commonly used passwords from the file “passwords.txt”
Sometimes it’s useful to read each line of a file separately and store them into a list. The example below reads the same list of common passwords but this time it splits the file into lines and stores each line of text into a list. The program then asks the user to enter a password and it checks if their password is in the list of commonly used passwords.
The most important part of the example above is line 3:
read()
returns a string containing all the data read from a file
.split("\n")
takes the file contents and splits it every time there’s a newline. It then returns a list of each line of the file
Reading CSV files
It can be really useful to organise the data you write to a file in a way that makes it easy to process in another program (such as a spreadsheet editor like Excel or LibreOffice Calc)
The easiest way to do this is to structure your data into a Comma Separated Values file (CSV). This just means your data is written to a text file but you write a comma ,
between every value and a new line \n
after every group of values.
The program below will load a list of scores that was generated from the previous tutorial about how to write CSV files with python. It loads names and scores from a file called scores.csv
:
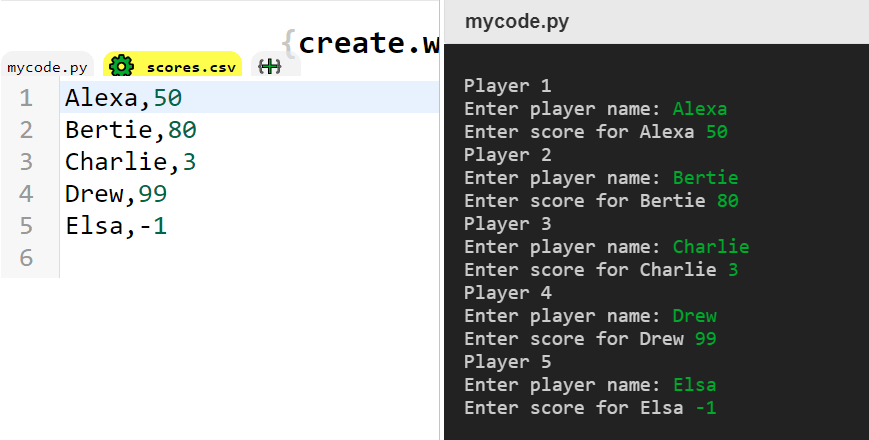
So far it only reads the data in as a list of strings (one string for each line). If we want to extract the name and score we have to loop through each line and split the data every time there is a ,
in order to make a 2D list:
The code above now reads in the data from the CSV file and displays each name and score as shown below:
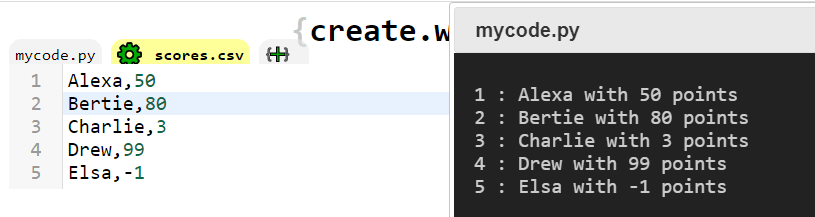
Page 1: Intro
Page 2: The theory: learn what you need to know as fast as possible.
Page 3: Try it: try out and adapt some working python code snippets.
Page 4: Debug it: Learn how to find and fix common mistakes.
Page 5: Extend it: Choose a project idea to use your newfound python skills.